Overview
Introduction
Welcome to Greenback! The Greenback Platform allows you to build modern web and mobile applications with high-def itemized financial data.
High-def itemized financial data includes all the details of a transaction that are missing on a typical credit card or bank feed. Rather than just an amount of money and a brief description, high-def itemized data includes the line items, vendor/supplier information, postal addresses, tax (sales, VAT, GST), payment instrument details, transaction type, invoice number, and more. In a nutshell, the fully detailed data that represents a GAAP-compliant financial transaction. Greenback provides a common data model and developer-friendly method to sync or extract data using various methods.
APIs
Let's get acquainted with the Greenback products.
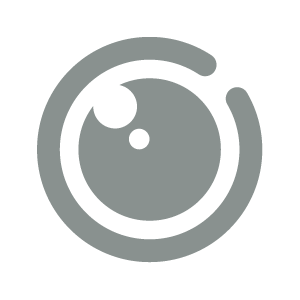
Convert images and documents into structured transaction data.
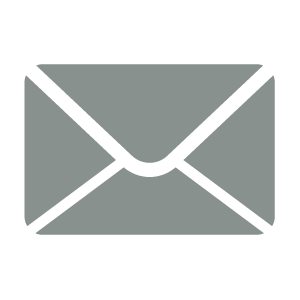
Convert RFC822 emails, including attachments and embedded links into structured transaction data.
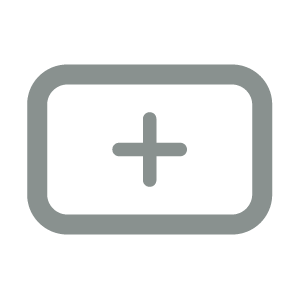
The complete transaction platform with support for rich connected accounts and Mailbox connectivity.
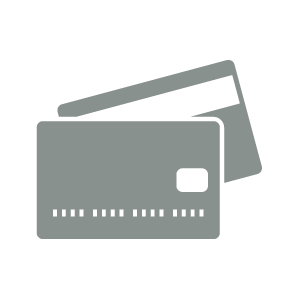
Sync, view and manage connected and non-connected accounts.
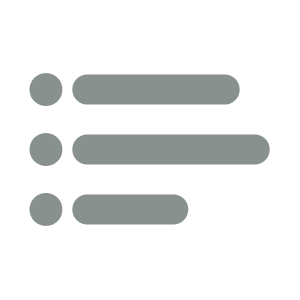
Search, view, and manage rich detailed transaction data.
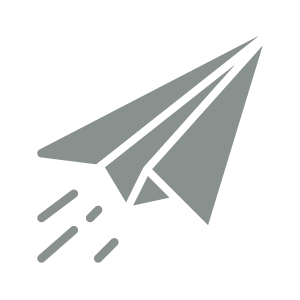
Export transactions to general ledgers.
You’ll find comprehensive information for integrating with the Greenback API endpoints and graphical UI plugin components. For assistance please contact our support group by email at support@greenback.com.
The Greenback API is organized around REST. Our API has predictable resource-oriented URLs, accepts JSON-encoded request bodies, returns JSON-encoded responses, and uses standard HTTP response codes, authentication, and verbs.
API |
---|
Vision |
Mailbox |
Connect |
Account |
Transaction |
Export |
Software Kits and Demos
The Greenback GitHub page will include any open source implementations and demos of the Greenback platform, data models, and APIs.
Project | URL |
---|---|
Greenback Kit for Java | https://github.com/greenback-inc/greenback-java |
Greenback Android Demo | https://github.com/greenback-inc/greenback-android-demo |
For example, here is a simple demo Android application of the Greenback Platform and our Vision API to extract receipt data from a photo taken by a mobile phone camera.
Get Access Token
Interested in our API? Contact our Business Development team via ping@greenback.com for more information.
Authentication
All requests to the Greenback API must include an access token (API key) in order to be authenticated. Any number of access tokens can be generated by Greenback that are associated with your overall organization and are unique to your account.
Your API keys carry many privileges, so be sure to keep them secure! Do not share your secret API keys in publicly accessible areas such as GitHub, client-side code, and so forth.
Once you have acquired your access token (API key) you simply add it to your request as an HTTP Authorization header with a Bearer scope. For example, if your access token was 123456789
then to send a request via curl.
Test Authentication
curl -v -H "Authorization: Bearer 123456789" https://api.greenback.com/v2/users/me
HTTP Request
GET /v2/users/me HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 123456789
User-Agent: curl/7.47.0
Accept: */*
All API requests must be made over HTTPS. Calls made over plain HTTP will fail. API requests without authentication will also fail.
Hosts
Greenback supports two environments: Production and Sandbox. The two environments are entirely separate at Greenback and your access tokens (API keys) will only be valid in the environment they were issued in.
Environment | Host |
---|---|
Sandbox | https://sandbox-api.greenback.com |
Production | https://api.greenback.com |
Versioning
Greenback versions every API endpoint. If major changes are released, and they break backwards compatibility, Greenback will ship new versioned API endpoints, while maintaining previous versions to allow for smooth migrations.
Within a released version (e.g. v2), Greenback may add new fields and enum values. It's critical in your implementation to ignore unknown properties while processing our JSON to maintain compatibility with our API over time. Most major JSON library across languages supports this concept. For example, in Java, the popular Jackson library will ignore unknown properties with a simple annotation at the top of your class file.
Many JSON processing libraries will NOT easily support unknown "enum" values. Please ensure your JSON library supports mapping unknown enum values or perhaps consider processing enums as strings.
Sample Java Class
@JsonIgnoreProperties(ignoreUnknown = true)
public class Response {
private String error;
public String getError() {
return error;
}
public void setError(String error) {
this.error = error;
}
}
Responses
All Greenback endpoints that return JSON-encoded data use a common outer response object. We recommend you use the same strategy in your implementation and use a single base object or class to represent any Greenback response.
JSON Response
{
"error" : { ... },
"pagination" : { ... },
"value" : { ... },
"values" : [ ... ]
}
The Response Object
Field | Type | Description |
---|---|---|
error | object | If the request failed then this represents the error. See the error object for more details. |
pagination | object | If the response is from a bulk/list endpoint then this object will help you to paginate thru the results. See the pagination object for more details. |
value | object | If the response is from an endpoint that returns a single value. The value varies by the endpoint. |
values | object[] | If the response is from a bulk/list endpoint this is an array of those values. The object within the array varies by the endpoint. |
Errors
Greenback uses conventional HTTP response codes to indicate the success or failure of an API request. In general: Codes in the 2xx
range indicate success. Codes in the 4xx
range indicate an error that failed given the information provided (e.g., a required parameter was omitted, a vision request failed, etc.). Codes in the 5xx
range indicate an error with Greenback's servers (these are rare).
In addition to the HTTP status code, the API returns a JSON-encoded response with a top-level error
object with detailed information.
HTTP Response
HTTP/1.1 401 Unauthorized
Content-Type: application/json; charset=utf-8
Content-Length: 114
Date: Fri, 19 Apr 2019 01:49:43 GMT
{
"error" : {
"code" : 10002,
"message" : "Access token rejected",
"category" : "unauthorized"
}
}
The Error Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
code | integer | The underlying Greenback error code. |
message | string | A user-friendly message describing what went wrong. |
category | string | The class of errors to simplify error handling. For example, unauthorized represents any authentication failure. Values may include: bad_request , unauthorized , not_found_or_bad_request , forbidden , not_found , not_found_or_forbidden , internal_error , temporary_error |
Pagination
Many top-level API resources have support for bulk fetches via "list" API methods. For instance, you can list transactions, list connects, etc. These list API methods share a common structure, where the first page of results will return a common pagination
object that includes information about the entire result as well as an optional next
field.
JSON Response
{
"pagination" : {
"count" : 100,
"total_count" : 3116,
"next" : "o-100,tc-3116"
}
}
If the next
field is present that means there is another page of data available. The next
value will become the cursor
field on your next request, and so on.
Some endpoints can paginate in both directions (forward and reverse). For these endpoints, the pagination
object will contain next
initially and also include previous
and current
fields as you fetch various pages. If these values are present, then the page can support both fetching data in either direction.
JSON Response
{
"pagination" : {
"count" : 100,
"total_count" : 3116,
"previous" : "o-100,tc-3116",
"next" : "o-300,tc-3116",
"current" : "o-200,tc-3116"
}
}
The Pagination Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
count | long | The count of records in the current result (page) of data. |
total_count | long | (Optional) The total count of records for the current search/query of data. |
next | string | (Optional) The cursor value to fetch the next result (page) of data. All list/batch endpoints support forward fetching of data. The value of this text should be passed thru as-is since future versions of this string may be obfuscated. |
previous | string | (Optional) The cursor value to fetch the previous result (page) of data. Not all list/batch endpoints support reverse fetching of data. The value of this text should be passed thru as-is since future versions of this string may be obfuscated. |
current | string | (Optional) The cursor value of the current result (page) of data. The value of this text should be passed thru as-is since future versions of this string may be obfuscated. |
Use Cases
The Greenback platform is a powerful pre-accounting system to help connect, sync, and manage financial transactions. There are a number of use cases in how businesses leverage our platform, here are just a few along with the various endpoints and steps they would entail.
Greenback for Business Custom Transactions
Greenback for Business user needs to integrate and post transactions from a source Greenback does not yet support, or perhaps from their own internal system. They want to leverage Greenback's other Software-as-a-Service features such as other integrations, reporting, exports, and automations such as Transforms.
Contact Greenback Sales and request a Personal Access Token. If your billing subscription allows API access then a Personal Access Token will allow you to utilize most aspects of our published API, such as manual transactions.
Create a shoebox account to store and organize the custom Transactions you need to create and update. If you are a Greenback for Business user, and you're logged into our web application, please visit Connect a Shoebox to create a Shoebox account.
Note the
account_id
of your new account in your browser URL such as "https://www.greenback.com/app/accounts/xyz123456" which means the account_id is xyz123456.Use the Create Transaction endpoint, using the shoebox account_id to create custom Transactions.
Login to Greenback for Business and interact with your Transactions as though Greenback had synced them directly from one of its supported integrations. All features are available with these transactions such as Transforms, Advanced Mappings, Exports, etc.
Financial Institution Receipt or Invoice Extraction
Financial institutions (e.g. bank or credit card providers) can leverage Greenback to build a user-friendly interface to allow uploads of receipts, invoices, or bills in various document formats for extraction.
Use the Create Vision endpoint to upload photos or PDFs for processing. Greenback will process the photo, extract detailed financial transaction data, and return a response within seconds.
Use the Create Message endpoint to upload RFC822 mime messages (emails) for processing. Greenback will process the email and extract detailed financial transaction data and return a response within seconds.
Leverage the rich extracted information for receipt matching or other features within your own application.
Financial Institution Exports to Accounting
Financial institutions (e.g. bank or credit card providers) can leverage Greenback to build a user-friendly interface to directly reconcile and export financial data from a bank or credit card portal to an accounting system such as QuickBooks or Xero.
Exports are stateful operations, which means the transaction you want to export must exist on Greenback prior to starting the export process. Use the Create Account endpoint to directly create a shoebox account type. Due to enforcing uniqueness of transactions per-account, it is a best practice to create an account to mirror the account of data you are trying to export.
Create and update transactions on Greenback that represent the data you want to export. Use the Create Transaction and Update Transaction endpoints as needed.
Use the Get Transaction Export Intent endpoint to present your user with matches or the export form to help answer questions required to complete the export.
Use the Get Transaction Export to help present information about the state of what was exported.
Common Types
The Greenback API uses many of the same types across endpoints.
DateTime
Many API resources include a DateTime type as one or more fields. Greenback supports standard ISO date and time formatting of this type, typically in the UTC (Zulu) timezone such as "2021-04-06T01:02:03.456Z".
For example, in Java, the java.time.Instant
type is an excellent choice to represent a Greenback DateTime.
Accounting Method Enum
Represents an accounting method in various objects within Greenback. See our reference Java implementation for more details.
Value | Description |
---|---|
cash | Cash basis accounting where the transaction was accrued at the same time as it was paid. |
accrual | Accrual accounting where the transaction was accrued and the cash payments for it were paid at a different time. |
pseudo_accrual | A method used to workaround accounting systems that do not support true accrual, where the payments are treated as separate transactions to mimic an accrual transaction as closely as possible. |
The Processing Status Enum
Many API resources have a status
field which represents the processing status of that resource. This is common across all endpoints. See our reference Java implementation for more details.
JSON Response
{
"status" : "error",
"error" : {
"code" : 10000,
"message" : "Access token rejected",
"category" : "unauthorized"
}
}
Value | Description |
---|---|
pending | Item was accepted for processing and is waiting to be processed. |
processing | Item is processing. |
success | Item was successfully processed and its results are ready. |
error | Item failed during processing. The item will usually include an error with more details about what failed. |
The Attachment Object
See our reference Java implementation for more details.
The Attachment Object
{
"rel_id": "sto_jYOeO",
"name": "Message.pdf",
"media_type": "application/pdf",
"reference_id": "msg_dbaa5f52-e0df-4a12-9c00-56811f4a939b_pdf",
"why": "original",
"size": 182464,
"md5": "e882c89c98e502969722110a2adf0128"
}
Field | Type | Description |
---|---|---|
rel_id | string | The unique identifier of the attachment relative to the other attachments. This is not a globally unique identifier. |
name | string | (Optional) The name of the attachment. |
media_type | string | (Optional) The standard media (MIME) type of the attachment such as application/pdf or image/png . |
reference_id | string | (Optional) A custom field for referencing something else. |
why | enum | (Optional) The reason an attachment exists. Values include: original , vat_invoice , and extra |
size | long | (Optional) The size (in bytes) of the attachment. |
md5 | string | (Optional) The MD5 hash of the attachment. |
The Email Address Object
See our reference Java implementation for more details.
The Email Address Object
{
"name": "Henry Ford",
"address": "henry@example.com"
}
Field | Type | Description |
---|---|---|
name | string | The name of the email address. |
address | string | The address (e.g. user@example.com) |
The Line Item Object
Represents a line item on Greenback. See our reference Java implementation for more details.
The Line Item Object
{
"grn": "it:asin:B0087RVIOU",
"name": "Sugar In The Raw 200 Count",
"uri": "https://www.amazon.com/gp/product/B0087RVIOU/ref=ppx_od_dt_b_asin_title_s00?ie=UTF8&psc=1",
"quantity": 2,
"unit_price": 16.47,
"amount": 32.94
}
Field | Type | Description |
---|---|---|
grn | string | (Optional) A namespaced, unique identifier of the product/service purchased or sold. The format follows a URN syntax of it:namespace:value . It will always start with it which indicates it is an item GRN. The namespace helps identify what kind value is present. The namespace includes: vs , sku , var , gb , and asin . Please see item grn for more details. |
alt_grns | string[] | (Optional) An array of alternative grn values. |
name | string | (Optional) The name of the item. |
description | string | (Optional) The description of the item (e.g. variations). |
uri | string | (Optional) A URL of the product/service. |
quantity | double | (Optional) The quantity purchased or sold. |
unit_price | double | (Optional) The price per unit. |
amount | double | (Optional) The total amount (e.g. quantity * unit_price). |
Item GRN
A Greenback Resource Number to identify what item was sold or purchased. The format follows a URN syntax of it:namespace:value
. It will always start with it
which indicates it is an item
GRN. The namespace helps identify what kind value is present.
Type | Description |
---|---|
vs | A vendor specific code such as a product identifier. |
sku | A stock keeping unit defined by the seller. Many accounting programs track items primarily by SKU. |
var | A variation identifier defined by the seller. A variation usually includes the length, color, etc. of an item. |
gb | A Greenback defined value usually used for standard items on our platform such as Payment Processing Fees, Marketplace Facilitator Taxes, etc. |
asin | An Amazon product identifier |
The Contact Object
Represents a party or entity that is a party to a transaction (e.g. the buyer or seller). See our reference Java implementation for more details.
{
"id" : "lBOp8mArOd",
"name" : "Domino's Pizza",
"domain" : "dominos.com"
}
Field | Type | Description |
---|---|---|
id | string | A unique identifier of the contact on Greenback. |
name | string | The name of the contact. |
domain | string | (Optional) The DNS domain of the contact if they are well-known such as amazon.com or ebay.com. |
The Form Object
Represents a Form (user input needed) on Greenback. See our reference Java implementation for more details.
The Form Object
{
"title": "Enter your 6th & Washington credentials",
"fields": [
{
"type": "text",
"name": "username",
"label": "Username",
"required": true
},
{
"type": "password",
"name": "password",
"label": "Password",
"required": true
},
{
"type": "button",
"name": "submit",
"label": "Connect"
}
]
}
Field | Type | Description |
---|---|---|
fields | object[] | The array of form input fields. |
title | string | (Optional) A title of the form that can be displayed to the user. |
description | string | (Optional) An overview of the form that can be displayed to the user. |
expires_at | datetime | (Optional) A date and time when the form expires. Only used on forms that are time-limited such as answering a 2-factor question. |
The Form Field Object
Represents an input within a Form. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
type | enum | The type of form input which generally mirrors HTML5 types. Values include: text , email , password , hidden , button , select |
name | string | (Optional) The name of the input. This mirrors HTML5 forms as this is the name of the key for the parameter that will be collected. |
label | string | (Optional) The text to display of what the input is for. This mirrors an HTML form label of a field. |
value | string | (Optional) A value to pre-populate in a form input. |
required | boolean | (Optional) Whether the input is required or not. If not present, defaults to false. |
help | string | (Optional) A helpful tip to display to user related to the form input. |
pattern | string | (Optional) A regex pattern to be used for validating the form input. |
pattern_message | string | (Optional) A message to display if the regex pattern is not validated. |
error | string | (Optional) If an error occurs during authorization related to a form input. |
options | object[] | (Optional) The array of select options if the field type is select . |
The Form Select Option Object
Represents an option for a select field within a Form. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
value | string | (Optional) The value of the select option |
text | string | (Optional) The text to display of what the select option is for |
tertiary_text | string | (Optional) Additional text to display alongside the text. Usually enclosing this in parenthese next to the text is a simple way to render this. input. |
selected | boolean | (Optional) Whether the option is currently selected |
The Postal Address Object
Represents a physical, geographic place on Greenback. Usually is a standard postal address, but can sometimes simply be a lat/long, or raw text describing the location. See our reference Java implementation for more details.
The Postal Address Object
{
"name": "Greenback, Inc.",
"line1": "307 W 6th St",
"line2": "Suite 209",
"city": "Royal Oak",
"region_code": "MI",
"postal_code": "48067",
"country_code": "us"
}
Or
{
"location": {
"lat": 40.76745,
"lon": -73.86358
},
"raw_address": "Grand Central Pkwy, New York, NY"
}
Field | Type | Description |
---|---|---|
name | string | (Optional) The name of the recipient. |
line1 | string | (Optional) The first line of the address. |
line2 | string | (Optional) The second line of the address. |
city | string | (Optional) The city of the address. |
region_code | string | (Optional) The region (e.g. state or province) of the address. |
postal_code | long | (Optional) The postal code. |
country_code | string | (Optional) The ISO-3166 country code of the address. |
location | geopoint | (Optional) A geographic point consisting of a lat/long. |
raw_address | string | (Optional) If a well-known address cannot be parsed, this represents the raw address text from the transaction source. |
Vision
The Greenback Vision API uses advanced AI (artificial intelligence), natural language, and machine learning techniques, trained on its massive and proprietary transaction dataset, to simplify the extraction of structured transaction data from real world photos and documents.
With the Greenback Vision API you can quickly and easily build web or mobile applications that can convert images (PNG/JPG) or documents (Microsoft Word/HTML/PDF) that contain receipts, bills, invoices, statements, and other types into structured and annotated data. The API is designed to process documents in near real-time, generally averaging around 1-3 seconds for industry-leading accuracy and speed. Greenback will return the recognized text, bounding boxes, annotations, and structured data. To get started, simply upload an image or document to the Greenback Vision API endpoint.
Create Vision | POST /v2/visions |
Get Vision | GET /v2/visions/{vision_id} |
Get Vision Attachment | GET /v2/visions/{vision_id}/attachments/{attachment_id} |
Get Vision Rasterized Image | GET /v2/visions/{vision_id}/rasterized |
Get Vision Annotated Image | GET /v2/visions/{vision_id}/annotated |
Vision Examples (JPEG, PNG)
File (URL) | Notes |
---|---|
7eleven.com_receipt_20190621.jpg | Standard convenience store receipt |
acehardware.com_receipt_20190623.jpg | Tall, small text, with watermarks |
bonefishgrill.com_receipt_20181214.jpg | Faded and old |
chipotle.com_receipt_20200115.jpg | Crumpled, wrinkled, skewed various angles |
dollargeneral.com_receipt_20190913.jpg | Significant skewing, complex line detection |
homedepot.com_receipt_20181027.jpg | Crumpled, wrinkled, skewed various angles |
indianatollroad_receipt_20181129-1155.jpg | Standard toll road receipt |
jimbradys_receipt_20190314.jpg | Multiple documents related to each other |
marathon.com_receipt_20181129.jpg | Standard fuel receipt |
mobil.com_receipt-sideways_20181224.jpg | 90 degree orientation |
sanfordrecycle_receipt_20190913.jpg | |
tractorcenter_receipt_20190913.jpg | Yellow tones, full page invoice |
traderjoes.com_receipt_20170809.png | Low quality scan, complex line detection |
Vision Examples (PDF)
File (URL) | Notes |
---|---|
business.comcast.com_invoice_20171118.pdf | Internet utility bill |
digitalocean.com_invoice_20190501.pdf | Cloud hosting bill |
disney.com_receipt_20190303.pdf | Point-of-sale receipt using monospaced text |
elliotelectric.com_invoice_20181019.pdf | Wholesale supplier bill |
fedex.com_receipt_20191013.pdf | Malformed PDF with text containing invalid unicode character mappings |
highandorange_receipt_20190905.pdf | PDF containing only an embedded image |
honorsone.com_invoice_20180824.pdf | Invoice generated by QuickBooks desktop |
sendgrid.com_invoice_20190101.pdf | Software-as-a-service bill |
wayfair.com_receipt_20200805.pdf | Each character is an image rather than true text |
xero.com_invoice-example.pdf | Invoice generated by Xero |
Vision Examples (Microsoft Word)
File (URL) | Notes |
---|---|
seafood-express_invoice_20190412.docx | Modern Microsoft Word format |
seafood-express_invoice_20190412.doc | Legacy Microsoft Word format (e.g. 1997) |
Vision Types
The Vision Object
The Vision Object
{
"id" : "kg1DMpNKXA",
"name" : "receipt.png",
"status" : "success",
"created_at" : "2019-04-02T14:25:49.299Z",
"updated_at" : "2019-04-02T14:25:49.421Z",
"attachments" : [ {
"rel_id" : "rs_1tydV5YSlh9bOS8fVHt9k",
"media_type" : "image/png",
"size" : 29566,
"md5" : "ff12c0a4a15f17049c09054f52f14be3"
} ],
"error" : { ... },
"transaction_matcher" : {
"transacted_ats" : [ {
"start" : "2018-12-14T01:00:00.000Z",
"end" : "2018-12-16T23:00:00.000Z"
}, {
"start" : "2019-01-14T01:00:00.000Z",
"end" : "2019-01-16T23:00:00.000Z"
} ],
"amounts" : [ {
"value" : 15.0
} ]
},
"annotations" : {
"full_text" : {
"text" : "Greenback, Inc. Receipt\nPaid to\nPaid by\nReceipt number\n2574-8279\nGreenback, Inc.\nHenry Ford\nInvoice number\n6C95218-0012\n307 W 6th St, Suite 208\nDate paid\nDecember 15, 2018\nRoyal Oak MI 48067\nPayment method: Visa – 7990\nUnited States\n+1 248-952-8217\nsupport@greenback.com\n$15.00 paid on December 15, 2018\nDescription\nQty\nUnit price\nAmount\nDEC 15, 2018 – JAN 15, 2019\nEarly Adopter Plan\n1\n$15.00\n$15.00\nSubtotal\n$15.00\nAmount paid\n$15.00\nQuestions? Contact Greenback, Inc. at support@greenback.com or call at +1 248-952-8217 or take a survey @ www.greenback.com/survey :-)\n2574-8279 – Page 1 of 1\n"
},
"temporals" : [ {
"text" : "12/01/2018",
"date" : "2018-12-01",
"granularity" : "day"
}, {
"text" : "JAN 15, 2019",
"date" : "2019-01-15",
"granularity" : "day"
} ],
"moneys" : [ {
"text" : "$15.00",
"symbol" : "$",
"amount" : 15.0,
"currency_code" : "USD"
} ],
"tenders" : [ {
"method" : "visa",
"ends_with" : "7990"
} ],
"urls" : [ {
"text" : "www.greenback.com/survey",
"url" : "http://www.greenback.com/survey",
"domain" : "greenback.com"
} ],
"emails" : [ {
"text" : "support@greenback.com",
"domain" : "greenback.com"
} ]
}
}
The vision object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
id | string | The unique identifier of the Vision object. |
name | string | The name of the vision object which is equal to the name of the file that was uploaded. |
status | enum | The processing status of the vision request. See the Processing Status enum for more details. |
created_at | datetime | ISO 8601 timestamp of when the vision object was created. |
updated_at | datetime | ISO 8601 timestamp of when the vision object was last updated. |
error | object | If the status is error then this will be present and is the error that occurred during processing. See the error object for more details. |
attachments | object[] | An array of attachment objects for any images or documents that are part of the Vision object. See the attachment object for more details. |
annotations | object | If the status is success then this includes all of the annotations extracted from the uploaded image or document. See the annotations object for more details. |
transaction_matcher | object | If the status is success then this includes our suggested criteria for matching to a transaction in another system. See the transaction matcher object for more details. |
The Transaction Matcher Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
transacted_ats | object[] | An array of interval objects that include a start and end datetime for a range of dates that the transaction may have occurred at. The ranges are calculated using the extracted data, context, granularity, and other heuristics to help you find a matching transaction. |
amounts | object[] | An array of monetary amount objects that represent possible transaction values such as the total or sub-total (e.g. authorized pre-tip amount). The amounts are calculated using the extracted data, context, and other heuristics to help you find a matching transaction. |
The Annotations Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
full_text | object | The full text annotation of the processed Vision image or document. See the full text annotation object for more details. |
temporals | array | An array of temporal annotations of the processed Vision object. See the temporal annotation object for more details. |
moneys | array | An array of money annotations of the processed Vision object. See the money annotation object for more details. |
tenders | array | An array of tender (payment instrument) annotations of the processed Vision object. See the tender annotation object for more details. |
emails | array | An array of email annotations of the processed Vision object. See the email annotation object for more details. |
urls | array | An array of url annotations of the processed Vision object. See the url annotation object for more details. |
The Full Text Annotation Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
text | string | The full text of the image or document. The ordering of the text generally follows how a human would read the image or document, but may be re-ordered for optimized processing into structured data. |
The Temporal Annotation Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
text | string | The original, raw text of the recognized date or time within the image or document. |
date | localdate | (Optional) The local date that was recognized. |
time | localtime | (Optional) The local time that was recognized. |
granularity | enum | The granularity of the date or time that was recognized. For example, if only a date was extracted the granularity would be to the day , whereas if a date and time such as "8:32 AM" were extracted then granularity would be to the minute . Values include: year , month , day , hour , minute , second , and millisecond . |
The Money Annotation Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
text | string | The original, raw text of the recognized money (monetary) amount within the image or document. |
amount | double | The amount of money. |
symbol | string | (Optional) The symbol of the money amount. |
currency_code | enum | (Optional) The ISO 3-letter currency code of the money amount. |
The Tender Annotation Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
method | enum | (Optional) The method of tender (payment). Values include: visa , mastercard , amex , discover , and cash |
ends_with | string | (Optional) The tail (e.g. last 4) of the tender. |
The URL Annotation Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
text | string | The original, raw text of the recognized URL within the image or document. |
url | string | A normalized, well-formed version of the URL that mimics how a browser would interpret it. |
domain | string | The top private domain of the URL host. For example, if the URL was either www.example.co.uk/survey or simply example.co.uk/survey then the domain value would be example.co.uk in either case. |
The Email Annotation Object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
text | string | The original, raw text of the recognized email address within the image or document. |
domain | string | The top private domain of the email address. For example, if the email address was either henry@em.example.co.uk or simply henry@example.co.uk then the domain value would be example.co.uk in either case. |
Create Vision
Upload an image (JPEG/PNG) or document (PDF) to create a new vision request. Both synchronous and asynchronous processing of your request is supported.
In synchronous mode Greenback will accept your request, process it, and return a result in a single step. In asynchronous mode Greenback will accept your request and return a unique identifier. The unique identifier can be used with the Get Vision endpoint to poll for updated status.
The HTTP request consists of multipart form data with a file parameter named file
.
Send Request (synchronous mode)
curl -v -X POST -H 'Authorization: Bearer 1234567890' -F 'file=@receipt.png' https://api.greenback.com/v2/visions
HTTP Request
POST /v2/visions HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Content-Length: 29604
Content-Type: multipart/form-data; boundary=b22c56d1-607a-4c13-abce-02c671633123
--b22c56d1-607a-4c13-abce-02c671633123
Content-Disposition: form-data; name="file"; filename="receipt.png"
Content-Type: application/octet-stream
Content-Length: 29566
<binary data>
--b22c56d1-607a-4c13-abce-02c671633123--
JSON Response (synchronous mode)
{
"value" : {
"id" : "kg1DMpNKXA",
"name" : "receipt.png",
"status" : "success",
"created_at" : "2019-04-02T14:25:49.299Z",
"updated_at" : "2019-04-02T14:25:49.421Z",
"attachments" : [ {
"rel_id" : "rs_1tydV5YSlh9bOS8fVHt9k",
"media_type" : "image/png",
"size" : 29566,
"md5" : "ff12c0a4a15f17049c09054f52f14be3"
} ],
"transaction_matcher" : { ... },
"annotations" : { ... }
}
}
Or JSON Response (asynchronous mode)
{
"value" : {
"id" : "kg1DMpNKXA",
"name" : "receipt.png",
"status" : "processing",
"created_at" : "2019-04-02T14:25:49.299Z",
"updated_at" : "2019-04-02T14:25:49.421Z",
"attachments" : [ {
"rel_id" : "rs_1tydV5YSlh9bOS8fVHt9k",
"media_type" : "image/png",
"size" : 29566,
"md5" : "ff12c0a4a15f17049c09054f52f14be3"
} ]
}
}
Endpoint
POST /v2/visions?async={async}
Field | Type | Description |
---|---|---|
async | boolean | (Optional) Either synchronous or asynchronous processing mode. Defaults to false. |
Request
Standard multipart form data with a multipart/form-data
content type. The image or document will be uploaded as the file
parameter.
Field | Type | Description |
---|---|---|
file | file | The image or document to upload. The name of the file and its file extension is how Greenback detects its mime-type. |
Response
A JSON-encoded response is returned with either an error or a Vision object. See the vision object for more details.
Get Vision
Get a Vision object by its unique identifier.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/visions/kg1DMpNKXA
HTTP Request
GET /v2/visions/kg1DMpNKXA HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response (synchronous mode)
{
"value" : {
"id" : "kg1DMpNKXA",
"name" : "receipt.png",
"status" : "success",
"created_at" : "2019-04-02T14:25:49.299Z",
"updated_at" : "2019-04-02T14:25:49.421Z",
"attachments" : [ {
"rel_id" : "rs_1tydV5YSlh9bOS8fVHt9k",
"media_type" : "image/png",
"size" : 29566,
"md5" : "ff12c0a4a15f17049c09054f52f14be3"
} ],
"transaction_matcher" : { ... },
"annotations" : { ... }
}
}
Endpoint
GET /v2/visions/{vision_id}
Field | Type | Description |
---|---|---|
vision_id | string | The unique identifier of the Vision object. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Vision object. See the vision object for more details.
Get Vision Attachment
Get the original document that was submitted as the Vision object. This attachment can be accessed by its relative unique identifier.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/visions/kg1DMpNKXA/attachments/rs_1tydV5YSlh9bOS8fVHt9k
HTTP Request
GET /v2/visions/kg1DMpNKXA/attachments/rs_1tydV5YSlh9bOS8fVHt9k HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: */*
If-None-Match: 123456789456879874556fdfdf
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: image/png
ETag: "ff12c0a4a15f17049c09054f52f14be3"
Content-Length: 29566
<binary data here>
Endpoint
GET /v2/visions/{vision_id}/attachments/{attachment_id}
Field | Type | Description |
---|---|---|
vision_id | string | The unique identifier of the Vision object. |
attachment_id | string | The relative, unique identifier of attachment. This is the rel_id value in the attachment object. |
Request
Standard GET request will contain an empty body.
Response
The binary image or document.
Get Vision Rasterized Image
Get a rasterized image of the Vision object. If the original document was already a rasterized image (e.g. PNG or JPEG) then the response will usually return that image data. If the original document was not originally rasterized (e.g. a PDF) then the response will return a rasterized (rendered) version of that document. The width and height of the rasterized image will match the dimensions used for annotating and processing of the Vision request.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/visions/kg1DMpNKXA/rasterized
HTTP Request
GET /v2/visions/kg1DMpNKXA/rasterized HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: */*
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: image/png
Content-Length: 29566
<binary data here>
Endpoint
GET /v2/visions/{vision_id}/rasterized
Field | Type | Description |
---|---|---|
vision_id | string | The unique identifier of the Vision object. |
Request
Standard GET request will contain an empty body.
Response
The binary image or document.
Get Vision Annotated Image
Get an annotated image of the Vision object. The response will be an SVG (image/svg+xml) image with multiple layers representing the image-layer
and annotation-layer
layers as well as optionally the symbol-layer
and text-layer
if your application is permitted to request them.
You can embed this SVG into an HTML document and utilize standard CSS styling for customizing how it looks as well as listen for events (e.g. hovers). Since we expect this SVG to be possibly directly included as part of an HTML document, the id
of all elements are prefixed with gbvl-
for layers, gbva-
for annotations, gbvs-
for symbols, and gbvt-
for texts.
<svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" viewBox="0 0 702 1262" version="1.1">
<defs>
<style type="text/css"><![CDATA[#gbvl-annotation-layer .annotation {fill: #33ff00; fill-opacity: 0.2; stroke: #33ff00; stroke-opacity: 0.2; stroke-width: 2.0;}]]></style>
</defs>
<g id="gbvl-image-layer" data-layer="image">
<image x="0" y="0" width="702" height="1262" xlink:href="data:image/png;base64,iVBORw0KGgoAAAANSUh..."/>
</g>
<g id="gbvl-annotation-layer" data-layer="annotation">
<rect id="gbva-0-0" class="annotation url" x="214" y="1171" width="237" height="31"/>
<rect id="gbva-0-1" class="annotation phone" x="324" y="111" width="186" height="35"/>
<rect id="gbva-0-2" class="annotation money" x="517" y="860" width="75" height="35"/>
<rect id="gbva-0-3" class="annotation money" x="520" y="885" width="71" height="39"/>
<rect id="gbva-0-4" class="annotation money" x="508" y="912" width="84" height="42"/>
<rect id="gbva-0-9" class="annotation money" x="498" y="387" width="67" height="30"/>
<rect id="gbva-0-10" class="annotation money" x="496" y="604" width="71" height="30"/>
<rect id="gbva-0-23" class="annotation money" x="484" y="688" width="83" height="29"/>
<rect id="gbva-0-24" class="annotation money" x="484" y="769" width="81" height="26"/>
<rect id="gbva-0-25" class="annotation place" x="357" y="88" width="115" height="34"/>
<rect id="gbva-0-26" class="annotation temporal" x="233" y="174" width="75" height="24"/>
<rect id="gbva-0-27" class="annotation temporal" x="360" y="175" width="74" height="24"/>
<rect id="gbva-0-28" class="annotation temporal" x="57" y="1048" width="252" height="34"/>
</g>
</svg>
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/visions/kg1DMpNKXA/annotated
HTTP Request
GET /v2/visions/kg1DMpNKXA/annotated HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: */*
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: image/svg+xml
Content-Length: 29566
<binary data here>
Endpoint
GET /v2/visions/{vision_id}/annotated
Field | Type | Description |
---|---|---|
vision_id | string | The unique identifier of the Vision object. |
Request
Standard GET request will contain an empty body.
Response
The binary image or document.
Mailbox
The Greenback Mailbox API uses advanced AI (artificial intelligence), natural language, and machine learning techniques to simplify the extraction and display of structured data from real world emails and attachments.
With the Greenback Mailbox API, you can quickly convert original or forwarded RFC822 emails, including attachments and embedded URLs into structured transaction data. Greenback leverages its Vision and Connect technologies to power the core of its Mailbox API, then combines that with information extracted from both inside and outside the email to build the richest and most-complete representation of the data. The API is designed to process emails in near real-time, generally averaging around 2-6 seconds for industry-leading accuracy and speed. Additional Mailbox APIs help power compelling and secure display of original or forwarded email content inside your own product. To get started, simply upload the email to the Greenback Mailbox API endpoint.
Create Message | POST /v2/messages |
Get Message | GET /v2/messages/{message_id} |
Get Message Attachment | GET /v2/messages/{message_id}/attachments/{attachment_id} |
Get Message HTML | GET /v2/messages/{message_id}/html |
Get Message PDF | GET /v2/messages/{message_id}/pdf |
Mailbox Examples (RFC822)
File (URL) | Notes |
---|---|
apple.com_receipt-icloud-20190117.msg | HTML body of email is a cloud service receipt |
bestbuy.com_receipt_20190123.msg | HTML body of email is a point-of-sale receipt |
consumersenergy.com_bill_20200303.msg | HTML body of email is a utility bill |
disney.com_receipt-forwarded_20190302.msg | Forwarded email contains an attachment that is a point-of-sale receipt |
dominos.com_receipt_20181121.msg | HTML body of email is a online receipt |
dreamhost.com_receipt_20190806.msg | Text-only body of email is a billing statement |
homedepot.com_receipt_20200209.msg | HTML body and attachment are point-of-sale reciepts |
lowes.com_receipt_20191010.msg | Forwarded email contains an HTML body that is a point-of-sale receipt |
lyft.com_receipt_20191115.msg | HTML body of email is a ride receipt |
seamless.com_receipt_20181207.msg | HTML body of email is a food delivery order |
square.com_receipt_20171016.msg | HTML body of email is a point-of-sale receipt |
Quoted Emails (Forwards, Replies, etc.)
Greenback uses specialized and natural language processing to parse and recognize quoted email content such as when a user forwards or replies to an email. This support extends across even multiple forwards or replies. The quoted content and headers are useful to improve the accuracy of recognition and user-friendly display of email content. The Message object on the Greenback platform will include both the original and any quoted messages, along with their recognized properties. Some endpoints will optionally let you request either the unquoted or quoted versions when rendering HTML or PDFs.
By default, Greenback will prefer the innermost, quoted content when extracting structured transaction data, generating original PDFs, and more. This generally produces the most accurate results and beautiful user-friendly display.
Mailbox Types
The Message Object
The Message Object
{
"id" : "xa7qYR61K8",
"reference_id" : "4e562e56-6d49-448b-8b70-c3886479ed33",
"status" : "success",
"posted_at" : "2018-08-27T22:25:36.000Z",
"created_at" : "2019-05-20T05:01:51.137Z",
"updated_at" : "2019-05-20T05:01:55.184Z",
"flags" : [ "converted" ],
"size" : 60054,
"subject" : "Fwd: Receipt from Mr. Broast Rosemont",
"snippet" : "Receipt for $17.08 at Mr. Broast Rosemont on Aug 27 2018 at 5:25 PM. Square automatically sends receipts to the email address you used at any Square seller. Learn more Mr. Broast Rosemont How was your",
"full_text" : "... text of email and any attachments ...",
"quoted" : [ {
"subject" : "Receipt from Mr. Broast Rosemont",
"from" : {
"name" : "Mr. Broast Rosemont via Square",
"address" : "broast@reply2.squareup.com"
},
"to" : [ {
"address" : "henry@example.com"
} ],
"posted_at" : "2018-12-10T13:20:00.000Z"
} ],
"from" : {
"name" : "Henry Ford",
"address" : "henry@example.com"
},
"to" : [ {
"address" : "receipts@example.com"
} ],
"reply_to" : [ {
"name" : "Henry Ford",
"address" : "henry@example.com"
} ],
"attachments" : [ {
"rel_id" : "rs_1tydV5YSlh9bOS8fVHt9k",
"media_type" : "image/png",
"size" : 29566,
"md5" : "ff12c0a4a15f17049c09054f52f14be3"
} ],
"transactions" : [ {
"id" : "RJwaz6aEAW",
"type" : "purchase",
"currency_code" : "usd",
"reference_id" : "ar76blmRglPvfopGz1na08nuMF",
"account_id" : "RYj10MDEao",
"contact_id" : "rMOGneoBKW",
"transacted_at" : "2018-08-28T00:25:00.000Z",
"created_at" : "2019-05-20T05:01:55.177Z",
"updated_at" : "2019-05-20T05:01:55.184Z",
"flags" : [ "converted" ],
"attributes" : {
"message_reference_id" : "4e562e56-6d49-448b-8b70-c3886479ed33"
},
"attachments" : [ {
"reference_id" : "msg_4e562e56-6d49-448b-8b70-c3886479ed33_pdf",
"rel_id" : "sto_1nJ8Nj",
"name" : "Message.pdf",
"media_type" : "application/pdf",
"why" : "original",
"size" : 100990,
"md5" : "78948a92f36ec5e73e2e69fb61eee979"
} ],
"store_address" : {
"location" : {
"lat" : 41.994,
"lon" : -87.876
},
"raw_address" : "Mr. Broast Rosemont\n7104 N Manheim Rd\nRosemont, IL 60018"
},
"items" : [ {
"name" : "3 PC Tender Meal",
"description" : "Dipped in BBQ sauce",
"quantity" : 1.0,
"unit_price" : 5.0
}, {
"name" : "Beef Sandwich (Gyro Cheese Burger Meal)",
"quantity" : 1.0,
"unit_price" : 10.49
} ],
"payments" : [ {
"method" : "credit_card",
"network" : "visa",
"ends_with" : "1234"
} ],
"totals" : {
"sub" : 15.49,
"tax" : 1.59,
"items" : [ {
"type" : "tax",
"name" : "Sales Tax",
"amount" : 1.59
} ],
"grand" : 17.08
},
"contact" : {
"id" : "rMOGneoBKW",
"name" : "Mr. Broast Rosemont",
"account_id" : "RYj10MDEao"
}
} ]
}
The Message object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
id | string | The unique identifier of the Message object. |
reference_id | string | An additional unique identifier of the Message object used internally by Greenback. Prefer id as your reference. |
status | enum | The processing status of the message request. See the Processing Status enum for more details. |
posted_at | datetime | ISO 8601 timestamp of the message (e.g. the Date header field). |
created_at | datetime | ISO 8601 timestamp of when the message object was created on Greenback. |
updated_at | datetime | ISO 8601 timestamp of when the message object was last updated on Greenback. |
flags | enum[] | (Optional) Various flags about the message request. Values may include: converted and draft . |
size | long | The size in bytes of the message object data. |
subject | string | The subject of the message (e.g. the Subject header field). |
snippet | string | A short (up to 200 characters) description of the contents of the message. |
full_text | string | The extracted text (up to 10K characters) of the email plus any extracted text (up to 10K characters) from any attachments. |
quoted | object[] | (Optional) If the email was forwarded then this array represents the unquoted (unforwarded) partial of the email. Each item in the array represents a hierarchical unquoting of the original email. The ordering will match outermost to innermost. The last index of this array will be the innermost forwarded email content. Any property of the Message object may be included here. However, the most common will be subject , from , to , and posted_at |
from | object | (Optional) The sender of the email. See the email address object for more details. |
to | object[] | (Optional) An array of email address objects for the to of the email. See the email address object for more details. |
reply_to | object[] | (Optional) An array of email address objects for the reply-to of the email. See the email address object for more details. |
cc | object[] | (Optional) An array of email address objects for the cc of the email. See the email address object for more details. |
bcc | object[] | (Optional) An array of email address objects for the bcc of the email. See the email address object for more details. |
transactions | object[] | An array of transaction objects for any recognized or extracted transactions in the Message object. See the transaction object for more details. |
The Quoted Message Object
The Quoted Message Object
{
"posted_at" : "2018-08-27T22:25:36.000Z",
"subject" : "Fwd: Receipt from Mr. Broast Rosemont",
"from" : {
"name" : "Henry Ford",
"address" : "henry@example.com"
},
"to" : [ {
"address" : "receipts@example.com"
} ],
"reply_to" : [ {
"name" : "Henry Ford",
"address" : "henry@example.com"
} ]
}
The Quoted Message object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
posted_at | datetime | ISO 8601 timestamp of this object. |
subject | string | The subject of the message (e.g. the Subject header field). |
from | object | (Optional) The sender of the email. See the email address object for more details. |
to | object[] | (Optional) An array of email address objects for the to of the email. See the email address object for more details. |
reply_to | object[] | (Optional) An array of email address objects for the reply-to of the email. See the email address object for more details. |
cc | object[] | (Optional) An array of email address objects for the cc of the email. See the email address object for more details. |
bcc | object[] | (Optional) An array of email address objects for the bcc of the email. See the email address object for more details. |
Create Message
Upload a message (RFC822 compliant email) to create a new mailbox request. Asynchronous processing of your request is supported. Greenback will accept your request and return a unique identifier. The unique identifier can be used with the Get Message endpoint to poll for updated status.
Processing every email is a multistep process on the Greenback platform. The message object will receive updates as steps are completed. It is designed this way to enable you to display the message in your product before it is fully processed. Once the email is processed, you can then use its transactions
array for extracted receipts, bills, invoices, and other structured data.
The HTTP request consists of multipart form data with a file parameter named file
.
Send Request (synchronous mode)
curl -v -X POST -H 'Authorization: Bearer 1234567890' -F 'file=@email.msg' https://api.greenback.com/v2/messages
HTTP Request
POST /v2/messages HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Content-Length: 29604
Content-Type: multipart/form-data; boundary=b22c56d1-607a-4c13-abce-02c671633123
--b22c56d1-607a-4c13-abce-02c671633123
Content-Disposition: form-data; name="file"; filename="email.msg"
Content-Type: application/octet-stream
Content-Length: 29566
<binary data>
--b22c56d1-607a-4c13-abce-02c671633123--
JSON Response (synchronous mode)
{
"value" : {
"id" : "p3qg3VEqMe",
"reference_id" : "6ce9e332-d4e2-4f35-8d71-6c8b64790e6a",
"status" : "pending",
"posted_at" : "2018-11-27T23:08:49.000Z",
"created_at" : "2019-05-21T05:01:05.665Z",
"updated_at" : "2019-05-21T05:01:05.667Z",
"size" : 231654,
"subject" : "[Personal] Your Tuesday evening trip with Uber"
}
}
Endpoint
POST /v2/messages
Field | Type | Description |
---|
No query parameters.
Request
Standard multipart form data with a multipart/form-data
content type. The RFC822 email message will be uploaded as the file
parameter.
Field | Type | Description |
---|---|---|
file | file | The RFC822 email message to upload. The name of the file and its file extension is how Greenback detects its mime-type. |
Response
A JSON-encoded response is returned with either an error or a Message object. See the message object for more details.
Get Message
Get a Message object by its unique identifier. You will want to continue to poll for changes if the status is pending
or processing
since that means the processing of this message is not yet finalized.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/messages/kg1DMpNKXA
HTTP Request
GET /v2/messages/kg1DMpNKXA HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response (synchronous mode)
{
"value" : {
"id" : "xa7qYR61K8",
"reference_id" : "4e562e56-6d49-448b-8b70-c3886479ed33",
"status" : "success",
"posted_at" : "2018-08-27T22:25:36.000Z",
"created_at" : "2019-05-20T05:01:51.137Z",
"updated_at" : "2019-05-20T05:01:55.184Z",
"flags" : [ "converted" ],
"size" : 60054,
"subject" : "Fwd: Receipt from Mr. Broast Rosemont",
"snippet" : "Receipt for $17.08 at Mr. Broast Rosemont on Aug 27 2018 at 5:25 PM. Square automatically sends receipts to the email address you used at any Square seller. Learn more Mr. Broast Rosemont How was your",
"quoted" : [ {
"subject" : "Receipt from Mr. Broast Rosemont",
"from" : {
"name" : "Mr. Broast Rosemont via Square",
"address" : "broast@reply2.squareup.com"
},
"to" : [ {
"address" : "henry@example.com"
} ],
"posted_at" : "2018-12-10T13:20:00.000Z"
} ],
"from" : {
"name" : "Henry Ford",
"address" : "henry@example.com"
},
"to" : [ {
"address" : "receipts@example.com"
} ],
"reply_to" : [ {
"name" : "Henry Ford",
"address" : "henry@example.com"
} ],
"attachments" : [ {
"rel_id" : "rs_1tydV5YSlh9bOS8fVHt9k",
"media_type" : "image/png",
"size" : 29566,
"md5" : "ff12c0a4a15f17049c09054f52f14be3"
} ],
"transactions" : [ { ... } ]
}
}
Endpoint
GET /v2/messages/{message_id}
Field | Type | Description |
---|---|---|
message_id | string | The unique identifier of the Message object. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a message_id object. See the message object for more details.
Get Message Attachment
Get the attachment of a Message object by its relative unique identifier.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/messages/kg1DMpNKXA/attachments/rs_1tydV5YSlh9bOS8fVHt9k
HTTP Request
GET /v2/messages/kg1DMpNKXA/attachments/rs_1tydV5YSlh9bOS8fVHt9k HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: */*
If-None-Match: 123456789456879874556fdfdf
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: image/png
ETag: "ff12c0a4a15f17049c09054f52f14be3"
Content-Length: 29566
<binary data here>
Endpoint
GET /v2/messages/{message_id}/attachments/{attachment_id}
Field | Type | Description |
---|---|---|
message_id | string | The unique identifier of the Message object. |
attachment_id | string | The relative, unique identifier of attachment. This is the rel_id value in the attachment object. |
Request
Standard GET request will contain an empty body.
Response
The binary image or document.
Get Message HTML
Get an HTML (partial or complete) document that is ready for embedding inside another HTML document or rendering on its own. The HTML will be sanitized of javascript, inline css, and other potentially unsafe elements or attributes. If embeddable, then the outermost element will be a div
element, while non-embeddable will be a standard html
element. If the original email was text-only (no HTML part) then an HTML-ready version of that text will be returned here.
If the email represents one or more quoted emails (e.g. a forward) and Greenback was able to recognize and extract that quoted content, then you can optionally request the innermost unquoted content is returned.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/messages/kg1DMpNKXA/html
HTTP Request
GET /v2/messages/kg1DMpNKXA/html HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: */*
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: text/html; charset=uft-8
Content-Length: 29566
<html here>
Endpoint
GET /v2/messages/{message_id}/html?unquote={unquote}&embed={embed}
Field | Type | Description |
---|---|---|
message_id | string | The unique identifier of the Message object. |
unquote | boolean | (Optional) Whether the original content or the recognized innermost quoted content should be returned. Defaults to false. |
embed | boolean | (Optional) Whether the HTML should be embeddable inside another HTML document (outer element will be a div) or a well-formed, standalone HTML document (outer element will be html). Defaults to true. |
Request
Standard GET request will contain an empty body.
Response
The html (partial) document.
Get Message PDF
Get a PDF document of the rendered email message. The PDF will be similar to a user printing
an email message and saving it as a PDF. The binary data returned by this method should be saved locally since every call to this method will render a fresh PDF.
If the email represents one or more quoted emails (e.g. a forward) and Greenback was able to recognize and extract that quoted content, then you can optionally request the innermost unquoted content is returned.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/messages/kg1DMpNKXA/pdf
HTTP Request
GET /v2/messages/kg1DMpNKXA/pdf HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: */*
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: application/pdf
Content-Length: 29566
<binary data here>
Endpoint
GET /v2/messages/{message_id}/pdf?unquote={unquote}
Field | Type | Description |
---|---|---|
message_id | string | The unique identifier of the Message object. |
unquote | boolean | (Optional) Whether the original content or the recognized innermost quoted content should be used during PDF rendering. Defaults to false. |
Request
Standard GET request will contain an empty body.
Response
The PDF document of the rendered email message.
Connect
The Greenback Connect API will allow you to connect, authenticate and acquire transaction data directly from a growing list of supported integrations. The Connect API provides a unified method of connecting accounts using various authentication models including password-based web forms and oAuth redirect flows.
With the Connect API, you’ll be able to:
- Connect accounts and using both password-based and oAuth-based authentication.
- Handle additional Multi-factor authentication (2FA, Captcha, etc.) requests required from the account.
- Reconnect accounts that experience authentication failures.
- Sync transaction data from the account.
List Connects | GET /v2/connects |
Get Connect | GET /v2/connects/{connect_label} |
Begin Connect Intent | GET /v2/connects/{connect_label}/begin |
Reconnect Account Intent | GET /v2/accounts/{account_id}/reconnect |
Authorize Connect Intent | POST /v2/connect_intents/{token} |
Complete Connect Intent | POST /v2/connect_intents/{token}/complete |
Connecting Accounts (Connect workflow)
The Connect API includes a Connect Intent object that indicates the current state of a connect request and what action your application will need to take next in order to finalize connecting the account.
The Connect API is designed this way for two reasons: (1) flexibility with how each upstream provider handles authentication, and (2) it promotes the use of switch
statements to quickly handle all stages of the app implementation.
Greenback recommends handling the Connect Intent response from the various endpoints in a unified method in your app, with a switch statement to handle the action. You can view an example of how that may look in the code view (Java).
public Result handleConnect(ConnectIntent connectIntent) {
switch (connectIntent.getAction()) {
case OK: {
// return an HTTP 200 OK response
}
case REDIRECT: {
// use connectIntent.getRedirectUrl() to send a browser redirect back to user
}
case INPUT: {
// use connectIntent.getInput() to render an HTML5 form for user to complete
}
case ISSUED: {
// use connectIntent.getToken() to call Greenback 'Complete Connect' endpoint
// to create a new Account, then you could either use the returned Account in that response
// directly and display it to the user OR choose to use the returned Connect to recursively
// call this method again to leverage the ERROR or COMPLETED handlers.
}
case PROFILE: {
// multiple connected accounts were authorized by the user and one of them needs
// to be selected by the user
// use the connectIntent.getInput() to render an HTML5 form for user to complete
// and then process the input form data and call the complete endpoint again
// with the "parameters" field in the posted object
}
case CONFIRM: {
// a potential issue was detected such as a duplicate account being connected, a different
// account from what was being reconnected, and more. The confirmation stage lets the user
// confirm they want to proceed
// use the connectIntent.getConfirm().getMessage() to help the user decide
// the call the complete endpoint again with the "action" field in the posted object
}
case COMPLETED: {
// use connectIntent.getAccountId() or connectIntent.getAccount() to know which Account was created
// and display that to your app user
}
case ERROR: {
// use connectIntent.getError() to render an error for user to see
}
}
Typical Password Connect Flow
Display a list of available Connects to the user with the List Connects endpoint.
User selects a Connect. Display the Connect form to the user with the Begin Connect Intent endpoint. The Connect Intent object action will be returned to you with an action set to
input
.User fills in form and submits it. Send the form data to the Authorize Connect Intent endpoint.
Greenback authorizes the connection and tokenizes the authentication data. Greenback returns a Connect Intent object with the action set to
issued
and an authorization token. Send the authorization token to the Complete Connect Intent endpoint. Greenback creates a new account, starts the first sync of that account, and returns the account in the response. If a potential issue is detected where the complete could connect a duplicate account or a reconnect would connect a different account from what was originally connected, then its possible you'll need to process theconfirm
action.Poll the account periodically (e.g. every 2 seconds) to monitor the sync status and progress using the Get Account endpoint. Please note that you'll want to include the
syncs
value for theexpand
query parameter while getting the account, so that the syncs are included in the response.Some Connects require some sort of 2-factor authentication for the user to answer. Typically, this only happens on the initial sync of an account. While polling the account for sync status, you'll want to check the
input
field of the Sync object for whether a value is present. If a value is present, the user must supply answers, otherwise the sync will timeout after 3-5 minutes of inactivity.Once the account is synced, query the account for transactions with the List Transactions endpoint
Typical oAuth Connect Flow
Same as with password flow.
Same as with password flow.
Same as with password flow. Please note that most oAuth-based connects simply have empty forms that do not require any initial data to start. Your app will want to supply a
complete_url
value along with theparameters
field, so that Greenback knows where to redirect back to once the oauth process has completed.Greenback prepares for authorization and returns a
redirect
action back to your app along with aredirect_url
value. You must allow the user to visit this url in a browser so that they can authorize the connection directly with the upstream provider.User authorizes the connection. Upstream provider will then redirect back to Greenback. Greenback authorizes the connection and tokenizes the authentication data. Greenback then redirects the user back to your
complete_url
. Greenback will include atoken
parameter to your application endpoint.Send the authorization token to the Complete Connect Intent endpoint. Greenback creates a new account, starts the first sync of that account, and returns the account in the response.
Same as with password flow steps 5 onward.
Reconnecting Accounts (Reconnect workflow)
If an account experiences an authentication failure, the user will need to be prompted to re-connect the account. Greenback offers an optimized flow for re-connecting an account. For example, if the account used the password flow for connecting, the username will be pre-populated on the INPUT
stage form. Your app will want to call the Reconnect Account Intent endpoint.
Syncing Transactions from an Account
Once an account is connected and established, syncs need to be initiated for new data to be fetched from the source. By default, Greenback will begin the first, initial sync for each new Account in most cases. Syncs can also be triggered, on demand, by using the Create Account Sync endpoint.
Greenback is designed to potentially sync massive amounts of data that can take anywhere from seconds to hours to complete for a particular account. Only one sync can be pending on an Account at a time.
Syncs are also how authentication of an account is verified, 2-factor challenges are discovered, or other errors are uncovered that may need the user to help remedy. We suggest polling an Account on a frequent basis (every 2 to 3 seconds) when a sync is in-progress, so that these are relayed to your user in a timely fashion.
Testing Your Connect Workflow
Greenback includes a test e-commerce site https://www.6thandwashington.com as part of its platform to help test the connect flow, account syncing, and fetching of transactional data. To get started, visit https://www.6thandwashington.com/register and register for a free test account. Once registered, you can generate test purchases and generate the same kind of test data that Greenback can fetch from live integrations. Thus, by setting up the accounts you need, you can test invalid passwords or generate new transactions to validate your sync progress.
Most new applications are only permitted to connect to 6th & Washington during development. You can connect 6th & Washington by using any of the following connect labels in your application:
Connect Label | Description |
---|---|
saw |
Connects to https://www.6thandwashington.com using a password flow for authentication (no 2-factor). |
saw-mfa |
Connects to https://www.6thandwashington.com using a password flow for authentication with a 2-factor code required on first sign-in. |
saw-oauth |
Connects to https://www.6thandwashington.com using an OAuth flow for authentication. |
Connect Types
Connect Expands
Value | Description |
---|---|
card | Includes the associated connect card. |
The Connect Object
The Connect Object
{
"id": "Zz532dXNWJ",
"type": "merchant",
"tags": [
"expenses"
],
"name": "Amazon.com",
"label": "amazon",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/amazon.com.svg",
"contact_id": "lBOpD38eOd",
"card" : {
"description" : "Sync your personal or business expenses from Amazon.com.",
"help_url" : "http://example.com/more-help"
}
}
The Connect object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
id | string | The unique identifier of the Connect object. |
type | enum | The type of connect. See the connect type enum for more details. |
tags | string[] | (Optional) Features that a connect may provide such as sales , expenses , or possibly both. |
name | string | The name of the connect such as Amazon.com , Home Depot , Gmail , PayPal , etc. |
label | string | The unique human-readable identifier of the connect. Used as the primary identifier in other connect APIs. |
state | enum | The state of the connect. Connects can be stateful and the state may change periodically so we do not suggest caching them in your application. For example, if the upstream provider is performing maintenance, Greenback may return the maintenance state if we believe connects may fail until the upstream provider completes it maintenance window. Values include: active , maintenance , beta , and dev . |
logo_url | string | The URL of a logo that represents the connect. |
contact_id | string | (Optional) If the connect supplies data for a default, single entity this is the unique identifier of that Contact such as Amazon.com or Home Depot. |
created_at | datetime | ISO 8601 timestamp of when the connect object was created on Greenback. |
updated_at | datetime | ISO 8601 timestamp of when the connect object was last updated on Greenback. |
auth_strategy | enum | (Optional) The authentication strategy used to connect. Values may include default , none , or redirect . |
account_state | enum | (Optional) The initial state of the account to be connected. Typically this is used to help create "manual" accounts that are not active by default such as a Shoebox or Mailbox type of account. Please see the account object for more info. |
account_connection_state | enum | (Optional) The initial connection state of the account to be connected. Typically, if this state is "ok" the connect completion will not trigger an initial sync to be started. Please see the account object for more info. |
card | object | (Optional) A connect card containing additional information that may be useful to present to a user to assist them with connecting an account. Properties include description and help_url . |
The Connect Type Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
merchant | Connect to a merchant account for sales or expenses such as Amazon or Shopify. |
accounting | Connect to an accounting (general ledger) account such as QuickBooks Online or Xero. |
mailbox | Connect to a mailbox account primarily for expenses. |
shoebox | Connect to an account that typically is not syncable for manual transactions or generated thru vision requests. |
The Connect Intent Object
The Connect Intent Object
{
"token" : "7172jsjajC01-0ajayqmmss",
"action" : "input",
"connect_id" : "Zz532dXNWJ",
"created_at" : "2021-04-06T05:35:24.368Z",
"input" : {
"title" : "Enter your Amazon.com credentials",
"fields" : [ {
"type" : "email",
"name" : "username",
"label" : "Email",
"required" : true
}, {
"type" : "password",
"name" : "password",
"label" : "Password",
"required" : true
}, {
"type" : "button",
"name" : "submit",
"label" : "Connect"
} ]
}
}
The Connect Intent object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
token | string | The token to identify this connect attempt across authorization and completion. |
connect_id | string | The connect associated with this object. |
account_id | string | (Optional) The account associated with this object. If re-connecting an account, this will be populated initially, or if its a new account this will be only populated once the connect is completed. |
action | enum | The action to require of the connecting user to continue authorization. See the connect intent action enum for more details. |
input | object | (Optional) If the action is input or profile then this is the form to present to the user in order to continue authorization or completion. See the form object for more details. |
error | object | (Optional) If the action is error then this is the error to present to the user. See the error object for more details. |
redirect_url | string | (Optional) If the action is redirect then this represents the URL to redirect the user to in a browser to complete authorization. |
confirm | object | (Optional) If the action is confirm then this is the confirmation message to present to the user in order to continue completion. See the connect intent confirm object for more details. |
created_at | datetime | ISO 8601 timestamp of when this object was created. |
updated_at | datetime | (Optional) ISO 8601 timestamp of when this object was last updated. Usually nil on the initial connect intent response, but populated with a value once moved to authorized state. |
completed_at | datetime | (Optional) ISO 8601 timestamp of when this object was completed. |
connect | object | (Optional) The connect that is associated with this connect intent. See the connect object for more details. |
account | object | (Optional) The account that is being re-connected OR the newly created account upon completion. May only be populated in the final step unless an account is being reconnected. See the account object for more details. |
The Connect Intent Action Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
input | A form should be displayed asking questions. |
redirect | The user agent (browser) needs to visit a url to complete authentication. |
issued | The authorization was issued, ready for complete to the called. |
confirm | The authorization could be fully completed with confirmation. |
completed | The authorization is fully completed, account created (or re-connected). |
ok | A simple 200 OK response is required. |
error | An error occurred with the authorization. |
profile | Multiple accounts could be connected. The user must help choose one. |
The Connect Intent Confirm Object
The Connect Intent Confirm object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
message | string | The message to display to the user to either confirm or cancel. |
The Connect Intent Complete Action Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
confirm | Confirms the user would like to proceed |
cancel | Cancels the connect |
List Connects
List all connects available on the Greenback platform. A subset of information is returned for each connect compared to the Get Connect
endpoint which also allows you to request a card
expansion which includes more information about a connect.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/connects
HTTP Request
GET /v2/connects HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response (synchronous mode)
{
"pagination": {
"count": 38,
"total_count": 38
},
"values": [
{
"id": "vVxwolN1xz",
"type": "merchant",
"tags": [
"sales"
],
"name": "Amazon Seller (North America)",
"label": "amazon-seller-na",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/sellercentral.amazon.com.svg"
},
{
"id": "Zz532dXNWJ",
"type": "merchant",
"tags": [
"expenses"
],
"name": "Amazon.com",
"label": "amazon",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/amazon.com.svg",
"contact_id": "lBOpD38eOd"
},
{
"id": "KbWrVeQgWM",
"type": "merchant",
"tags": [
"expenses"
],
"name": "Best Buy",
"label": "bestbuy",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/bestbuy.com.svg",
"contact_id": "me5NQmwPgn"
},
{
"id": "lRxy6Kkw0k",
"type": "merchant",
"tags": [
"sales",
"expenses"
],
"name": "eBay",
"label": "ebay",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/ebay.com.svg",
"contact_id": "epOyv0eYgZ"
},
{
"id": "1g0QKb9J5Z",
"type": "merchant",
"tags": [
"sales",
"expenses"
],
"name": "Etsy",
"label": "etsy",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/etsy.com.svg",
"contact_id": "VAOlQk0Dg9"
},
{
"id": "3L0gJjVzxe",
"type": "mailbox",
"tags": [
"email"
],
"name": "Gmail",
"label": "gmail",
"state": "dev",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/gmail.google.com.svg"
},
{
"id": "3r063dJoWq",
"type": "merchant",
"tags": [
"expenses"
],
"name": "Home Depot",
"label": "home-depot",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/homedepot.com.svg",
"contact_id": "aVKdRwLmg1"
},
{
"id": "Gm0PMZ64Wj",
"type": "merchant",
"tags": [
"sales"
],
"name": "Shopify",
"label": "shopify",
"state": "active",
"logo_url": "https:\/\/www.greenback.com\/assets\/f\/logos\/shopify.com.svg",
"contact_id": "0NKjDZe8g4"
},
... many more
]
}
Endpoint
GET /v2/connects?expands={expands}&limit={limit}&cursor={cursor}&labels={labels}&states={states}&types={types}&min_created_at={min_created_at}&max_created_at={max_created_at}&min_updated_at={min_updated_at}&max_updated_at={max_updated_at}
Field | Type | Description |
---|---|---|
expands | string | (Optional) Additional associated objects to expand as part of the response. This is a placeholder for future expands, none are supported currently. |
limit | integer | (Optional) The limit for the number of connects to return for each page of results. Defaults to 100. |
cursor | string | (Optional) The cursor for the page of connects to return. See Pagination for more info. Defaults to none (first page of data). |
labels | string | (Optional) One or more connect labels to filter by. A comma-delimited list such as "saw,walmart-seller,quickbooks". Defaults to none (all) |
states | enum | (Optional) One or more connect states to filter by. A comma-delimited list such as "active,upcoming". See the Connect object for valid enum values. Defaults to all public states. |
types | enum | (Optional) One or more connect types to filter by. A comma-delimited list such as "merchant,accounting". See the Connect object for valid enum values. Defaults to none (all) |
min_created_at | datetime | (Optional) The starting (inclusive) date and time of the created_at value to filter by. Defaults to none (all) |
max_created_at | datetime | (Optional) The ending (exclusive) date and time of the created_at value to filter by. Defaults to none (all) |
min_updated_at | datetime | (Optional) The starting (inclusive) date and time of the updated_at value to filter by. Defaults to none (all) |
max_updated_at | datetime | (Optional) The ending (exclusive) date and time of the updated_at value to filter by. Defaults to none (all) |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with a paginated list of Connect objects. See pagination and the connect object for more details.
Get Connect
Get a connect object.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/connects/amazon
HTTP Request
GET /v2/connects/amazon HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response (synchronous mode)
{
"value" : {
"id" : "Zz532dXNWJ",
"type" : "merchant",
"tags" : [ "expenses" ],
"name" : "Amazon.com",
"label" : "amazon",
"state" : "active",
"logo_url" : "https://www.greenback.com/assets/f/logos/amazon.com.svg",
"contact_id" : "lBOpD38eOd",
"created_at" : "2021-04-02T23:34:06.000Z",
"updated_at" : "2021-04-02T23:34:06.000Z",
"auth_strategy" : "default",
"card" : {
"description" : "Sync your personal or business expenses from Amazon.com.",
"help_url" : "http://example.com/more-help"
}
}
}
Endpoint
GET /v2/connects/{connect_label}?expands={expands}
Field | Type | Description |
---|---|---|
connect_label | string | The unique identifier of the connect such as walmart-seller . |
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions to include. See connect expands for more details. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Connect object. See the connect object for more details.
Begin Connect Intent
Begin connecting a new account. This method creates a new Connect Intent that will provide a token to be used throughout the connection process.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/connects/amazon/begin
HTTP Request
GET /v2/connects/amazon/begin HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response (synchronous mode)
{
"value" : {
"token" : "S4Zq0IIFQbIVGAUBu7rnjPP3QMKyN6Zq",
"connect_id" : "Zz532dXNWJ",
"created_at" : "2021-04-06T05:31:36.866Z",
"action" : "input",
"input" : {
"title" : "Enter your Amazon.com credentials",
"fields" : [ {
"type" : "email",
"name" : "username",
"label" : "Email",
"required" : true
}, {
"type" : "password",
"name" : "password",
"label" : "Password",
"required" : true
}, {
"type" : "button",
"name" : "submit",
"label" : "Connect"
} ]
},
"connect" : {
"id" : "Zz532dXNWJ",
"type" : "merchant",
"tags" : [ "expenses" ],
"name" : "Amazon.com",
"label" : "amazon",
"state" : "active",
"logo_url" : "https://www.greenback.com/assets/f/logos/amazon.com.svg",
"contact_id" : "lBOpD38eOd",
"created_at" : "2021-04-02T23:34:06.000Z",
"updated_at" : "2021-04-02T23:34:06.000Z",
"auth_strategy" : "default"
}
}
}
Endpoint
GET /v2/connects/{connect_label}/begin
Field | Type | Description |
---|---|---|
connect_label | string | The unique identifier of the Connect object. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Connect Intent object. See the connect intent object for more details.
Reconnect Account Intent
Reconnect an existing account. This method creates a new Connect Intent that will provide a token to be used throughout the reconnection process.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/accounts/Azyajzy1s/reconnect
HTTP Request
GET /v2/accounts/Azyajzy1s/reconnect HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response (synchronous mode)
{
"value" : {
"token" : "S4Zq0IIFQbIVGAUBu7rnjPP3QMKyN6Zq",
"connect_id" : "Zz532dXNWJ",
"account_id" : "Azyajzy1s",
"created_at" : "2021-04-06T05:31:36.866Z",
"action" : "input",
"input" : {
"title" : "Enter your Amazon.com credentials",
"fields" : [ {
"type" : "email",
"name" : "username",
"label" : "Email",
"value" : "henry@example.com",
"required" : true
}, {
"type" : "password",
"name" : "password",
"label" : "Password",
"required" : true
}, {
"type" : "button",
"name" : "submit",
"label" : "Connect"
} ]
},
"connect" : {
"id" : "Zz532dXNWJ",
"type" : "merchant",
"tags" : [ "expenses" ],
"name" : "Amazon.com",
"label" : "amazon",
"state" : "active",
"logo_url" : "https://www.greenback.com/assets/f/logos/amazon.com.svg",
"contact_id" : "lBOpD38eOd",
"created_at" : "2021-04-02T23:34:06.000Z",
"updated_at" : "2021-04-02T23:34:06.000Z",
"auth_strategy" : "default"
}
}
}
Endpoint
GET /v2/accounts/{account_id}/reconnect
Field | Type | Description |
---|---|---|
account_id | string | This is the unique identifier of the account. Re-connecting an account will optimize the connection process when an account authorization is simply being updated or corrected. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Connect Intent object. See the connect intent object for more details.
Authorize Connect Intent
Authorize a connection by submitting form data and getting back the next step.
Send Request
curl -v -X POST -H 'Authorization: Bearer 1234567890' -H "Content-Type: application/json" -d '{ "parameters" : { "username": "henryford", "password": "test" } }' https://api.greenback.com/v2/connect_intents/S4Zq0IIFQbIVGAUBu7rnjPP3QMKyN6Zq
HTTP Request
POST /v2/connect_intents/lLkVlCrTENMcosgaSx44_R5-cb2ibUjS HTTP/1.1
Authorization: Bearer 1234567890
Content-Length: 62
Content-Type: application/json
{
"parameters" : {
"username": "henryford",
"password": "test"
}
}
JSON Response
{
"value" : {
"token" : "lLkVlCrTENMcosgaSx44_R5-cb2ibUjS",
"connect_id" : "jyWa9nJpW1",
"created_at" : "2021-04-06T14:48:58.266Z",
"updated_at" : "2021-04-06T14:48:58.632Z",
"action" : "issued",
"connect" : {
"id" : "jyWa9nJpW1",
"type" : "merchant",
"tags" : [ "expenses" ],
"name" : "6th & Washington",
"label" : "saw",
"state" : "dev",
"logo_url" : "https://www.greenback.com/assets/f/logos/6thandwashington.com.svg",
"contact_id" : "rMOGqlnV5W",
"created_at" : "2021-04-02T23:34:06.000Z",
"updated_at" : "2021-04-02T23:34:06.000Z",
"auth_strategy" : "default"
}
}
}
Endpoint
POST /v2/connect_intents/{token}
Field | Type | Description |
---|---|---|
token | string | The unique identifier of the Connect Intent object. |
Request
A JSON-encoded request as the HTML request body.
Field | Type | Description |
---|---|---|
parameters | object | A map of key-value pairs representing the input names and values. |
complete_url | string | If the connect flow includes a redirect_url this is the url Greenback wll redirect to your own app to handle the completion of the connect process. |
Response
A JSON-encoded response is returned with the updated Connect Intent object. Depending on what type of connect process is used, it is possible for the intent returned to be another input (e.g. a required field is missing and the form needs to be resubmitted), a redirect is required, or the connect was issued and a token is available. See the connect intent object for more details.
Complete Connect Intent
Completes a connection by consuming the issued authorization token, creating a new account, and optionally starting the first sync. The authorization token can be consumed just once and can only be assigned to a single account.
Send Request
curl -v -X POST -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/connect_intents/X2Kd5wlAD-dSXV8vRGa7ffZglw1U-g89/complete
HTTP Request
POST /v2/connect_intents/X2Kd5wlAD-dSXV8vRGa7ffZglw1U-g89/complete HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Content-Length: 0
Content-Type: application/json
JSON Response
{
"value" : {
"token" : "X2Kd5wlAD-dSXV8vRGa7ffZglw1U-g89",
"connect_id" : "jyWa9nJpW1",
"account_id" : "5abB3ldPaP",
"created_at" : "2021-04-06T14:55:20.028Z",
"updated_at" : "2021-04-06T14:55:20.514Z",
"completed_at" : "2021-04-06T14:55:20.514Z",
"action" : "completed",
"account" : {
"id" : "5abB3ldPaP",
"name" : "henryford",
"user_id" : "3ByO7xK7Xq",
"connect_id" : "jyWa9nJpW1",
"type" : "merchant",
"default_name" : "henryford",
"reference_id" : "henryford",
"connection_state" : "pending",
"state" : "active",
"consecutive_errors" : 0,
"created_at" : "2021-04-06T14:55:20.512Z",
"updated_at" : "2021-04-06T14:55:20.512Z"
},
"connect" : {
"id" : "jyWa9nJpW1",
"type" : "merchant",
"tags" : [ "expenses" ],
"name" : "6th & Washington",
"label" : "saw",
"state" : "dev",
"logo_url" : "https://www.greenback.com/assets/f/logos/6thandwashington.com.svg",
"contact_id" : "rMOGqlnV5W",
"created_at" : "2021-04-02T23:34:06.000Z",
"updated_at" : "2021-04-02T23:34:06.000Z",
"auth_strategy" : "default"
}
}
}
Profile Action
Greenback will detect possible multiple accounts that can be connected during the completion process. Some connections support authenticating multiple accounts in a single authorization. For example, Xero accounting connections will authenticate the user and then allow you to access any accounting files that they are a member of. After a connect intent is issued by Xero, if multiple accounting files are detected, Greenback may need to prompt the user to select which one they want to connect. This results in a profile
action, where the input
field of the connect intent object will contain a form that needs to be completed.
JSON Response
{
"value" : {
"token" : "omJXh7Oks131jds_IdQt4jzNMCZwK8gy",
"connect_id" : "jY5lJ3zV0O",
"created_at" : "2021-04-06T16:09:45.560Z",
"updated_at" : "2021-04-06T16:09:45.775Z",
"action" : "profile",
"input" : {
"title" : "Which Xero account would you like to connect?",
"fields" : [ {
"type" : "select",
"name" : "profile",
"label" : "Account",
"required" : true,
"options" : [ {
"value" : "",
"text" : "Select an account"
}, {
"value" : "a49f3ce8-3764-439f-98ac-d6ed33c7eab6",
"text" : "Henry Consulting LLC"
}, {
"value" : "6c18f354-9b97-4136-9ec8-38036a710ea9",
"text" : "Kangaroo Ltd"
}, {
"value" : "dfad74ac-8d43-4afe-92f9-b4c13d913e22",
"text" : "Widget, Inc."
} ]
} ]
},
"connect" : {
"id" : "jY5lJ3zV0O",
"type" : "accounting",
"tags" : [ "accounting" ],
"name" : "Xero",
"label" : "xero",
"state" : "active",
"logo_url" : "https://www.greenback.com/assets/f/logos/xero.com.svg",
"contact_id" : "8EOMqXJYOy",
"created_at" : "2021-04-02T23:34:13.000Z",
"updated_at" : "2021-04-02T23:34:13.000Z",
"auth_strategy" : "default"
}
}
}
Confirm Action
Greenback will detect possible unsafe scenarios during the completion process. For example, a user may try to connect the same account twice, in which case Greenback will need confirmation that it is okay to proceed with completing the account. If your call to the Complete Connect endpoint results in a stage of CONFIRM
you will need to relay the confirmation message to your user and then call the Complete Connect endpoint again with a query parameter of action
which represents which action you'd like Greenback to take on your behalf.
Please note that you can preemptively provide the action to take to avoid this intermediate stage of completing an account.
JSON Response
{
"value" : {
"token" : "X2Kd5wlAD-dSXV8vRGa7ffZglw1U-g89",
"connect_id" : "jyWa9nJpW1",
"created_at" : "2021-04-06T14:55:20.028Z",
"updated_at" : "2021-04-06T14:55:20.514Z",
"completed_at" : "2021-04-06T14:55:20.514Z",
"action" : "confirm",
"confirm" : {
"code" : 10121,
"message" : "You are attempting to connect a new 6th & Washington account 'hford', but you already have an existing account with the same identity. Do you want to proceed with re-connecting your existing account 'hford'?"
},
"connect" : {
"id" : "jyWa9nJpW1",
"type" : "merchant",
"tags" : [ "expenses" ],
"name" : "6th & Washington",
"label" : "saw",
"state" : "dev",
"logo_url" : "https://www.greenback.com/assets/f/logos/6thandwashington.com.svg",
"contact_id" : "rMOGqlnV5W",
"created_at" : "2021-04-02T23:34:06.000Z",
"updated_at" : "2021-04-02T23:34:06.000Z",
"auth_strategy" : "default"
}
}
}
Endpoint
POST /v2/connect_intents/{token}/complete
Field | Type | Description |
---|---|---|
token | string | The unique identifier of the Connect Intent object. |
Request
Field | Type | Description |
---|---|---|
parameters | object | A map of key-value pairs representing the input names and values. |
action | enum | The confirmation action to use if any potential issue is detected during the completion such as a duplicate account. Values include: confirm , cancel |
Response
A JSON-encoded response is returned with the updated Connect Intent object, its authorization, and the newly connected account or a confirmation message. See the connect intent object and the account object for more details.
Account
The Account API is for managing connected accounts, triggering syncs, and fetching sync status.
List Accounts | GET /v2/accounts |
Get Account | GET /v2/accounts/{account_id} |
Delete Account | DELETE /v2/accounts/{account_id} |
Create Sync | POST /v2/accounts/{account_id}/syncs |
Get Sync | GET /v2/syncs/{sync_id} |
Create Sync Input (for 2-Factor Flow) | POST /v2/syncs/{sync_id}/inputs |
Account Types
Account Expands
Value | Description |
---|---|
connect | Includes the associated connect. |
syncs | Includes the associated syncs (ok, last, and pending). |
The Account Object
The Account Object
{
"id": "exBKAywEad",
"default_name": "henryford",
"user_id": "kNgX7qmBzR",
"connect_id": "jyWa9nJpW1",
"type": "merchant",
"connection_state": "pending",
"state": "active",
"consecutive_errors": 0,
"created_at": "2020-01-27T19:49:40.381Z",
"updated_at": "2020-01-27T19:49:40.524Z",
"connect" : { ... },
"syncs" : {
"last" : {
"id" : "gbDWlA6yG0",
"triggered_by" : "web",
"type" : "default",
"reference_id" : "280efcf4-413e-11ea-a539-b97fd65bc3a9",
"message" : "OK",
"progress" : 1.0,
"created_at" : "2020-01-27T19:49:40.519Z",
"updated_at" : "2020-01-27T20:37:37.278Z"
}
}
}
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
id | string | The unique identifier of the Account object. |
default_name | string | The default name of the Account. Typically set from the upstream connection. |
overlay_name | string | The custom name of the Account, which is user-defined. Only set if an update to the name of this account is made by the API/user. |
name | string | Either the overlay_name if present, otherwise falls back to the default_name. This is a read-only property. |
user_id | string | The unique identifier of the associated User object for this account. |
connect_id | string | The unique identifier of the associated Connect object for this account. |
reference_id | string | A unique reference identifier for this account. Typically set from the upstream connection. |
type | enum | The type of Account. Values include: merchant , mailbox , accounting , shoebox . |
state | enum | The state of the Account. Values include: active , suspended , disconnected , deleted , manual . |
connection_state | enum | The connection state of the Account. Values include: pending , ok , failed , auth_failure . |
consecutive_errors | integer | The number of consecutive errors encountered during syncs. |
created_at | datetime | ISO 8601 timestamp of when the account object was created on Greenback. |
updated_at | datetime | ISO 8601 timestamp of when the account object was last updated on Greenback. |
connect | object | (Optional) If expanded this is the associated Connect object for this account. See the connect object for more details. |
syncs | object | (Optional) If expanded this is the associated Syncs object for this account. See the account syncs object for more details. |
The Account Syncs object
Field | Type | Description |
---|---|---|
last | object | (Optional) The last sync performed on this account regardless of whether it was successful or not. See the sync object for more details. |
ok | object | (Optional) The last sync performed on this account that was successful. See the sync object for more details. |
pending | object | (Optional) The current sync in-progress. See the sync object for more details. |
The Sync object
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
id | string | The unique identifier of the Sync object. |
account_id | string | The unique identifier of the associated Account object. |
triggered_by | string | The source of how the Sync was triggered. For example, web or api . |
type | enum | The type of Sync. Values include: default , indexing |
reference_id | string | A unique reference identifier for this sync. Used internally by Greenback. |
status | enum | The processing status of the sync request. See the Processing Status enum for more details. |
error | object | (Optional) If the status is error this is the error. See the error object for more details. |
message | string | A progress message to display to the User |
progress | double | A value between 0 and 1 representing the progress of the sync. |
input | object | (Optional) If the sync is currently paused waiting for input (e.g. 2-factor authentication) this is the form to display to the user to collect their answer. While an account is syncing, the sync should be polled periodically to see if the input field is present. If so, the user should be notified and prompted to complete it since there is typically a time limit. See the form object for more details. |
created_at | datetime | ISO 8601 timestamp of when the Sync object was created on Greenback. |
updated_at | datetime | ISO 8601 timestamp of when the Sync object was last updated on Greenback. |
Create Account
Creates a new account on Greenback. This allows you to skip the Connect process that is normally used for creating accounts, thereby allowing you to directly create an account. Directly created accounts are disconnected by their nature, since the Connect process is the only way to authorize them with an upstream integration. Creating accounts directly is useful for creating shoebox
accounts for manually created transactions.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' -XPOST -d @account.json https://api.greenback.com/v2/accounts
HTTP Request
POST /v2/accounts HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
Content-Length: 597
Content-Type: application/json
{
"connect_id" : "ey5GndNNWQ",
"reference_id" : "113-456-71234",
"default_name" : "My Account"
}
JSON Response
{
"value" : {
"id" : "7Y8o93kMa2",
"name" : "My Account",
"user_id" : "kNgX7qmBzR",
"connect_id" : "ey5GndNNWQ",
"type" : "shoebox",
"default_name" : "My Account",
"reference_id" : "gba-b58f9261be99406295f0c04ba21ce111",
"connection_state" : "ok",
"state" : "manual",
"consecutive_errors" : 0,
"created_at" : "2021-04-07T19:57:34.390Z",
"updated_at" : "2021-04-07T19:57:34.390Z"
}
}
Endpoint
POST /v2/accounts
Field | Type | Description |
---|
No query parameters.
Request
Standard post of JSON with a application/json
content type. The JSON consists of an Account object. See the account object for more details.
Response
A JSON-encoded response is returned with either an error or the new Account object. See the account object for more details.
List Accounts
List all accounts available on the Greenback platform for the authenticated user.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/accounts
HTTP Request
GET /v2/accounts HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"pagination": {
"count": 38,
"total_count": 38
},
"values" : [ {
"id" : "RYj10MDEao",
"name" : "Default Mailbox",
"user_id" : "3ByO7xK7Xq",
"connect_id" : "AEWBGYbP5k",
"type" : "mailbox",
"default_name" : "Default Mailbox",
"reference_id" : "gba-07daf79200ee410480ad42db2e18e780",
"connection_state" : "ok",
"state" : "active",
"consecutive_errors" : 0,
"created_at" : "2021-04-02T20:00:02.295Z",
"updated_at" : "2021-04-02T20:00:02.295Z"
}, {
"id" : "3xGlX4wVay",
"name" : "Default Vision",
"user_id" : "3ByO7xK7Xq",
"connect_id" : "ey5GndNNWQ",
"type" : "shoebox",
"default_name" : "Default Vision",
"reference_id" : "gba-691fc2c460b14c1d889f2b2b2d5fae8b",
"connection_state" : "ok",
"state" : "manual",
"consecutive_errors" : 0,
"created_at" : "2021-04-02T20:00:02.360Z",
"updated_at" : "2021-04-02T20:00:02.360Z"
}, {
"id" : "5abB3ldPaP",
"name" : "henryford",
"user_id" : "3ByO7xK7Xq",
"connect_id" : "jyWa9nJpW1",
"type" : "merchant",
"default_name" : "henryford",
"reference_id" : "henryford",
"connection_state" : "auth_failure",
"state" : "active",
"consecutive_errors" : 2,
"created_at" : "2021-04-06T14:55:20.512Z",
"updated_at" : "2021-04-06T15:56:12.697Z"
}, {
"id" : "ma4EwbqJY2",
"name" : "Widget, Inc.",
"user_id" : "3ByO7xK7Xq",
"connect_id" : "jY5lJ3zV0O",
"type" : "accounting",
"default_name" : "Widget, Inc.",
"reference_id" : "dfad74ac-8d43-4afe-92f9-b4c13d913e22",
"connection_state" : "ok",
"state" : "active",
"consecutive_errors" : 0,
"created_at" : "2021-04-06T16:18:34.525Z",
"updated_at" : "2021-04-06T16:18:37.726Z"
}
... many more
]
}
Endpoint
GET /v2/accounts?expands={expands}&limit={limit}&cursor={cursor}
Field | Type | Description |
---|---|---|
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See account expands for more details. |
limit | integer | (Optional) The limit for the number of objects to return for each page of results. Defaults to 100. |
cursor | string | (Optional) The cursor for the page of objects to return. See Pagination for more info. Defaults to none (first page of data). |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with a paginated list of Account objects. See pagination and the account object for more details.
Get Account
Get an account.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/accounts/exBKAywEad
HTTP Request
GET /v2/accounts/exBKAywEad HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value": {
"id": "exBKAywEad",
"user_id": "kNgX7qmBzR",
"connect_id": "jyWa9nJpW1",
"type": "merchant",
"default_name": "henryford",
"connection_state": "ok",
"state": "active",
"consecutive_errors": 0,
"created_at": "2020-01-27T19:49:40.381Z",
"updated_at": "2020-01-27T20:37:37.276Z",
"connect": { ... },
"syncs": {
"last": {
"id": "gbDWlA6yG0",
"triggered_by": "web",
"type": "default",
"reference_id": "280efcf4-413e-11ea-a539-b97fd65bc3a9",
"message": "Timeout",
"progress": 1,
"created_at": "2020-01-27T19:49:40.519Z",
"updated_at": "2020-01-27T20:37:37.278Z",
"status": "error",
"error": {
"code": 10303,
"message": "We encountered a timeout while syncing your account. Try again later.",
"category": "temporary_error"
}
}
}
}
}
Endpoint
GET /v2/accounts/{account_id}?expands={expands}
Field | Type | Description |
---|---|---|
account_id | string | The unique identifier of the Account object. |
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See account expands for more details. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or the Account object. See the account object for more details.
Delete Account
Delete an account.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' -XDELETE https://api.greenback.com/v2/accounts/exBKAywEad
HTTP Request
DELETE /v2/accounts/exBKAywEad HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value": {
"id": "exBKAywEad",
"user_id": "kNgX7qmBzR",
"connect_id": "jyWa9nJpW1",
"type": "merchant",
"default_name": "henryford",
"connection_state": "ok",
"state": "deleted",
"consecutive_errors": 0,
"created_at": "2020-01-27T19:49:40.381Z",
"updated_at": "2020-01-27T20:37:37.276Z"
}
}
Endpoint
DELETE /v2/accounts/{account_id}
Field | Type | Description |
---|---|---|
account_id | string | The unique identifier of the Account object. |
Request
Standard DELETE request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or the Account object. See the account object for more details.
Create Sync
Request an account sync.
Send Request
curl -v -X POST -H 'Authorization: Bearer 1234567890' -H 'Content-Type: application/json' -d '{"limit":"15", "from":"2019-12-27T21:40:54.427Z"}' https://api.greenback.com/v2/accounts/exBKAywEad/syncs
HTTP Request
POST /v2/accounts/exBKAywEad/syncs HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Content-Length: 0
Content-Type: application/json
(optional)
{
"limit": 15,
"from" : "2021-07-01T00:00:00.000Z"
}
JSON Response
{
"value": {
"id": "0gOvMjW7Xk",
"triggered_by": "web",
"type": "default",
"reference_id": "b21466cc-414d-11ea-b148-11c9c04b0867",
"message": "Pending",
"progress": 0,
"created_at": "2020-01-27T21:40:54.427Z",
"updated_at": "2020-01-27T21:40:54.427Z",
"status": "pending"
}
}
Endpoint
POST /v2/accounts/{account_id}/syncs
Field | Type | Description |
---|---|---|
account_id | string | The unique identifier of the Account object. |
Request
An empty HTTP request body or a JSON-encoded request representing the limit and from attributes for an advanced sync.
Response
A JSON-encoded response is returned with the Sync object. See the sync object for more details.
Get Sync
Get an account sync.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/syncs/LowVyqVzPQ
HTTP Request
GET /v2/syncs/LowVyqVzPQ HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value": {
"id": "LowVyqVzPQ",
"triggered_by": "web",
"type": "default",
"reference_id": "8dd5cbbb-414c-11ea-b148-11c9c04b0867",
"message": "OK",
"progress": 1,
"created_at": "2020-01-27T21:32:44.128Z",
"updated_at": "2020-01-27T21:32:52.541Z",
"summary": {},
"status": "success"
}
}
Endpoint
GET /v2/syncs/{sync_id}
Field | Type | Description |
---|---|---|
sync_id | string | The unique identifier of the Sync object. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or the Sync object. See the sync object for more details.
Create Sync Input
Updates a sync with inputs to help answer 2-factor questions.
Send Request
curl -v -X POST -H 'Authorization: Bearer 1234567890' -H 'Content-Type: application/json' -d '{ "parameters" : { "code": "123456" } }' https://api.greenback.com/v2/syncs/0gOvMjW7Xk/inputs
HTTP Request
POST /v2/syncs/0gOvMjW7Xk/inputs HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Content-Length: 44
Content-Type: application/json
{
"parameters": {
"code": "123456"
}
}
JSON Response
{
"value": {
"id": "0gOvMjW7Xk",
"triggered_by": "web",
"type": "default",
"reference_id": "b21466cc-414d-11ea-b148-11c9c04b0867",
"message": "Pending",
"progress": 0,
"created_at": "2020-01-27T21:40:54.427Z",
"updated_at": "2020-01-27T21:40:54.427Z",
"status": "pending"
}
}
Endpoint
POST /v2/syncs/{sync_id}/inputs
Field | Type | Description |
---|---|---|
sync_id | string | The unique identifier of the Sync object. |
Request
A JSON-encoded request representing the answers to the inputs that a sync is currently waiting for.
Response
A JSON-encoded response is returned with the Sync object. See the sync object for more details.
Transaction
The Transaction API is for querying transactions fetched or posted to an account.
Create Transaction | POST /v2/transactions |
Update Transaction | POST /v2/transactions/{transaction_id} |
List Transactions | GET /v2/transactions |
Get Transaction | GET /v2/transaction/{transaction_id} |
Get Transaction Attachment | GET /v2/transaction/{transaction_id}/attachments/{attachment_id} |
Transaction Data Model
The Greenback Transaction object is modeled to support both accrual and/or cash-basis accounting transactions. It has been carefully designed to normalize the way a transaction is modeled across a wide variety of platforms, specifically to support GAAP (Generally Accepted Accounting Principles) compliance.
The transaction object represents the overall properties of a sale or expense and is accrual by its nature, regardless of the type used. It is then paid or partially_paid when either payments
(for expense transaction types) or deposits
(for sale transaction types) are populated. Generally, the outer envelope of the transaction object are the accrual portion of a transaction, and the cash-basis accounting is accomplished by populating the payments or deposits fields.
While transaction data from most platforms can be converted into a Greenback Transaction in a straight forward one-to-one mapping, there are cases where the way some platforms model transactions that need more thought. For example, a refunded transaction is a good example. Many platforms will simply mark a refund as part of a state change of the same transaction. However, in GAAP, this is incorrect. If payment was received on the original transaction, the refund represents a new transaction that stands by itself. On Greenback, this transaction should be modeled as two separate transactions, with one representing the sale, and the other representing the refund.
Payments and Deposits
The payment or deposit on a transaction represents how the money/cash flowed to pay for it. In other words, what account did the funds clear through? Generally, on sales, the deposit represents what account the cash from the buyer is going to be directly credited to, which is usually an account that temporarily holds it. For example, if you processed the payment from the buyer via Stripe, you would want to populate the deposit to represent the Stripe account the funds would be temporarily held in (not the bank account Stripe may eventually deposit your funds to). On expenses, the payment represents what account the cash to the seller was directly sent from. For example, if you paid with a Visa credit card, you would want to populate the payment to represent this credit card.
On "cash in" transaction types (invoice, sales_receipt, or reimbursement transactions), the deposits
transaction field will represent if the transaction has been paid or partially paid.
On "cash out" transaction types (bill, purchase, or refund transactions), the payments
transaction field will represent if the transaction has been paid or partially paid.
On exports to an accounting system, Greenback leverages the method
and ends_with
fields of the payment object to help remember what account to map to or from. To be as flexible as possible with various systems, the method
field is limited to a small set of possible values. If a method is unavailable, Greenback generally recommends leveraging a concatenation of values to form the ends_with
field to help provide uniqueness, and setting the method to one of the possible values. Here are some examples:
Sale transaction processed with AfterPay/Klarna: method set to seller_account
and ends_with set to afterpay_12345
where 12345 is the known Klarna account number. If the account number is unknown, then an ends_with value of afterpay
may provide enough uniqueness for the specific user to accurately map to the accounting system.
Sale transaction processed with PayPal: method set to paypal
and ends_with set to paypal_A123B1234G
where A123B1234G is the known PayPal seller user id. If the seller user id is unknown, then an ends_with value of paypal
may provide enough uniqueness for the specific user to accurately map to the accounting system.
Please be careful with generating too many unique ends_with values if you are simply trying to differentiate between various instruments. For example, if you have various types of PayPal transactions such as express payments vs. standard payments, as long as the funds from those sales all end up in the same PayPal clearing account, make sure to use a single ends_with
value. You can leverage the name
field to provide more clarity or description of the payment without effecting how that payment is mapped to a target accounting system.
Transaction Types
Transaction Expands
Value | Description |
---|---|
links | Includes the associated links (to help find an associated message, or if a transaction was exported). |
contact | Includes the associated contact. |
account | Includes the associated account. |
The Transaction Object
The Transaction Object
{
"id" : "42d1YnO0oJ",
"type" : "purchase",
"currency_code" : "usd",
"reference_id" : "5560f493-a1c7-430e-8f15-1ca88b5fc055",
"transacted_at" : "2018-11-21T22:11:55.000Z",
"created_at" : "2019-05-24T05:08:14.952Z",
"updated_at" : "2019-05-24T05:08:14.956Z",
"flags" : [ "converted" ],
"attributes" : {
"display_reference_id" : "104512",
"ponum" : "123 Main"
},
"contact" : {
"id" : "lBOp8mArOd",
"name" : "Domino's Pizza",
"domain" : "dominos.com"
},
"attachments": [
{
"rel_id": "sto_1dguhF",
"media_type": "application/pdf",
"why": "original",
"size": 44985,
"md5": "bc4ceede6e882d859861a58c05624eb9"
}
],
"store_address": {
"line1" : "302 SOUTH WASHINGTON",
"line2" : "SUITE 212",
"city" : "Royal Oak",
"region_code" : "mi",
"postal_code" : "48067",
"country_code" : "us",
"raw_address": "302 SOUTH WASHINGTON \nROYAL OAK, MI 48067"
},
"items" : [ {
"name" : "Your Domino's Order",
"quantity" : 1.0,
"unit_price" : 21.19,
"amount" : 21.19
} ],
"travel" : { ... },
"payments" : [ {
"method" : "credit_card",
"network" : "visa",
"ends_with" : "1234"
} ],
"totals" : {
"sub" : 21.19,
"tax" : 1.24,
"items" : [ {
"type" : "tax",
"name" : "Sales Tax",
"amount" : 1.24
} ],
"grand" : 22.43
}
}
See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
id | string | The unique identifier of the Transaction object. |
type | enum | The type of the transaction. See the transaction type enum for more details. |
currency_code | enum | ISO 4217 currency code of the transaction. |
reference_id | string | A unique, stable reference identifier provided by the source of the transaction (e.g. an invoice number). This reference_id is scoped to the account the transaction is within and it must be unique within that account. If you need duplicate reference_id values, an alternative is to use the display_reference_id field instead. |
alt_reference_id | string | An alternative unique, stable reference identifier provided by the source of the transaction. This value is used in conjunction with the reference_id to help locate transactions. It has a number of uses, one main use case is to provide a method to migrate to a new reference_id value. |
display_reference_id | string | A non-unique reference identifier provided by the source of the transaction. When present this value supersedes the reference_id for public display and/or exporting. |
transacted_at | datetime | ISO 8601 timestamp of the transaction. |
created_at | datetime | ISO 8601 timestamp of when the transaction object was created on Greenback. |
updated_at | datetime | ISO 8601 timestamp of when the transaction object was last updated on Greenback. |
flags | enum[] | (Optional) Various flags about the transaction. Values may include: converted , draft , archived , trashed , duplicate , and exported . |
attributes | object | (Optional) A object of string keys and values of extra metadata about the transaction. |
contact | object | (Optional) The counter party of the transaction. For example, if this is your purchase, then this will represent who you purchased from (the seller). See the contact object for more details. |
attachments | object[] | (Optional) An array of attachment objects for any original, VAT receipts, or other documents attached to the transaction. See the attachment object for more details. |
status | object | (Optional) The status of the transaction. See the transaction status object for more details. |
store_address | object | (Optional) The postal address of the store where the transaction took place. See the postal address object for more details. |
billing_address | object | (Optional) The postal address of who was billed. See the postal address object for more details. |
shipping_address | object | (Optional) The postal address of where it was shipped. See the postal address object for more details. |
items | object[] | (Optional) An array of line items purchased or sold. See the line item object for more details. |
travel | object | (Optional) If the transaction is related to travel (e.g. Lyft or Uber) then this represents what travel occurred. See the travel object for more details. |
exchange_rates | object[] | (Optional) An array of exchange rate objects that represents what rate was/is used from the transaction currency to the exchange rate currency. See the exchange rate object for more details. |
payments | object[] | (Optional) An array of payment objects that represents the source/origin of funds for a transaction. See the payment object or payments and deposits for more details. |
deposits | object[] | (Optional) An array of payment objects that represents the target/destination of funds for a transaction. See the payment object or payments and deposits for more details. |
totals | object | (Optional) The total amounts of the transaction. See the transaction totals object for more details. |
The Transaction Type Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
purchase | Purchase (expense) |
bill | Bill (expense) |
reimbursement | Reimbursement of a previous expense (a negative expense) |
sales_receipt | Sales receipt (sale) |
invoice | Invoice (sale) |
refund | Refund of a previous sale (a negative sale) |
The Transaction Status Object
Represents a transaction status. See our reference Java implementation for more details.
{
"state" : "paid",
"updated_at" : "2019-05-24T01:12:23.000Z"
}
Field | Type | Description |
---|---|---|
state | enum | The financial state of the transaction. See the transaction state enum for more details. |
message | string | (Optional) A description of the current status. Primarily useful for the open state to describe why the transaction is still open. |
updated_at | datetime | (Optional) ISO 8601 timestamp of the state was last updated. |
The Transaction State Enum
Represents a transaction status. See our reference Java implementation for more details.
Value | Description |
---|---|
open | Transaction is not yet ready for exporting to an accounting system. Useful for scenarios such as exchange rates, tax amounts, or other financial data that is not complete enough, where the transaction needs more time to complete. |
unpaid | The transaction is finalized, but not yet paid. |
partially_paid | The transaction has been partially paid. More payments are expected. |
paid | The transaction has been fully paid. |
voided | The transaction was canceled (before any payments were received). If a payment was received then please note that should be entered as another transaction to represent a refund. |
The Payment Object
Represents a flow of cash/money in or out such as from a credit card or to a bank account. Can either be a payment (cash out) or a deposit (cash in). For a more general overview of how to use this object, see payments and deposits for more info. See our reference Java implementation for more details.
{
"method" : "credit_card",
"network" : "visa",
"ends_with" : "1234",
"amount" : 12.24,
"name" : "My Business Visa",
"paid_at" : "2019-05-24T01:12:23.000Z"
}
Field | Type | Description |
---|---|---|
method | enum | The method of payment (or deposit). On sales, the most common method will be seller_account . On expenses, the most common method will be credit_card . See the payment method enum for more details. |
network | enum | (Optional) The network of payment (or deposit) related to the method. See the payment method enum for more details. |
reference_id | string | (Optional) An identifier (typically from the source) to help reference the payment or deposit. |
ends_with | string | (Optional) The last (or full) part of an account number such as the last 4 digits of a credit card. This is an important value to help Greenback remember what account to map a payment or deposit to in an accounting system. In many cases if you know the full account number, supply it here instead of the last few characters. See payments and deposits for more details. |
amount | double | (Optional) The amount of the payment or deposit. |
name | string | (Optional) The name or description of the account to help the user identify which one was used. |
paid_at | datetime | (Optional) ISO 8601 timestamp of the payment or deposit. |
The Payment Method Enum
Represents a method of payment (or deposit). See our reference Java implementation for more details.
Value | Description |
---|---|
seller_account | An account, similar to a bank account, where funds are temporarily held on e-commerce or payment platforms such as Stripe, Etsy, AfterPay, Klarna, etc. The most widely used method on sales-related or payment platforms. |
credit_card | A credit card (or a general card if debit vs. credit not necessarily known). |
debit_card | A debit card |
reward | Rewards points |
paypal | PayPal |
gift_card | A gift card (which was previously purchased earlier in time). This specifically helps to debit against a liability account where the original gift card purchase was deposited to. |
google_wallet | (Legacy) A google wallet, although Greenback recommends using a credit_card method more generically. |
money_transfer | (Legacy) A money transfer, although Greenback recommends using a cash method more generically. |
cash | A cash payment where funds would be directly wired/deposited to a bank account. |
store_credit | |
check | (Legacy) A check, although Greenback recommends using a cash method more generically. |
apple_pay | (Legacy) A apple pay, although Greenback recommends using a credit_card method more generically. |
money_order | (Legacy) A money transfer, although Greenback recommends using a cash method more generically. |
bank_account | A direct transfer to/from a bank account, similar to cash. |
The Payment Network Enum
Represents a network of payment (or deposit) related to the method. Currently used with credit_card
or debit_card
payment methods. See our reference Java implementation for more details.
Value | Description |
---|---|
visa | Visa credit or debit card |
mastercard | MasterCard credit or debit card |
amex | American Express credit or debit card |
discover | Discover credit or debit card |
The Exchange Rate Object
Represents an exchange rate from one currency to another. See our reference Java implementation for more details.
{
"currency_code" : "eur",
"rate" : 1.32,
"type" : "presentment"
}
Field | Type | Description |
---|---|---|
currency_code | string | The ISO currency code of the exchange rate. |
rate | double | The rate of exchange from the source to this exchange rate currency code. |
type | enum | (Optional) The type of exchange rate. Values may include: presentment , settlement , or estimated . |
The Travel Object
Represents a type of travel occurred on the Greenback platform. Included as part of a transaction so that a map of the various legs of travel can be rendered along with the transaction itself.
{
"type": "car",
"legs": [
{
"depart_at": "2019-04-25T12:13:05.000Z",
"arrive_at": "2019-04-25T12:42:18.000Z",
"depart_address": {
"location": {
"lat": 40.76506,
"lon": -73.97508
},
"raw_address": "54 W 59 St, New York, NY 10019"
},
"arrive_address": {
"location": {
"lat": 40.76745,
"lon": -73.86358
},
"raw_address": "Grand Central Pkwy, New York, NY"
},
"distance": 16737.178,
"ticket_class": "Lyft"
}
]
}
The Transaction Totals Object
A summary of the totals of a transaction. It consists of 3 parts: the subtotal, non-sub totals, and the grand total. The subtotal is a sum of the line items. Non-sub totals are amounts such as tax, shipping, fees, discounts, etc. The grand is the subtotal and non-sub totals and is the final amount. See our reference Java implementation for more details.
The Transaction Totals Object
{
"sub" : 21.19,
"tax" : 1.24,
"items" : [ {
"type" : "tax",
"name" : "Sales Tax",
"amount" : 1.24
} ],
"grand" : 22.43
}
Field | Type | Description |
---|---|---|
sub | double | The total line item amount of the transaction. |
tax | double | The total tax amount of the transaction. |
ship | double | The total shipping amount of the transaction. |
fee | double | The total fee amount of the transaction. |
tip | double | The total tip amount of the transaction. |
discount | double | The total discount amount of the transaction. |
other | double | The total other amount of the transaction. |
grand | double | The grand total of the transaction which is sub plus all other non-sub totals. |
items | object[] | An array of totals item objects that itemize what tax, shipping, fee, tip, discount, or other items are present. |
Create Transaction
Creates a new transaction on Greenback. Most transactions on Greenback are automatically created as part of another process such as a connected account (and syncing transactions from an upstream source), or through the Vision or Message APIs. This endpoint allows the application to create their own set of Transactions.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' -XPOST -d @transaction.json https://api.greenback.com/v2/transactions
HTTP Request
POST /v2/transactions HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
Content-Length: 597
Content-Type: application/json
{
"reference_id" : "113-456-71234",
"type" : "sales_receipt",
"currency_code" : "usd",
"account_id" : "za5ygRK6am",
"items" : [ {
"grn" : "it:sku:WIDGET-1",
"name" : "Widget",
"quantity" : 2.0,
"unit_price" : 1.12,
"amount" : 2.24
} ],
"deposits" : [ {
"method" : "paypal",
"amount" : 3.74,
"paid_at" : "2021-04-02T01:02:03.456Z"
} ],
"totals" : {
"sub" : 2.24,
"tax" : 1.5,
"items" : [ {
"type" : "tax",
"name" : "Tax",
"amount" : 1.5
} ],
"grand" : 3.74
},
"transacted_at" : "2021-04-01T01:02:03.456Z"
}
JSON Response
{
"value" : {
"id" : "e5waKyOnRv",
"type" : "sales_receipt",
"currency_code" : "usd",
"reference_id" : "113-456-71234",
"account_id" : "za5ygRK6am",
"transacted_at" : "2021-04-01T01:02:03.456Z",
"created_at" : "2021-04-07T04:04:09.996Z",
"updated_at" : "2021-04-07T04:04:09.998Z",
"flags" : [ "manual" ],
"items" : [ {
"grn" : "it:sku:WIDGET-1",
"name" : "Widget",
"quantity" : 2.0,
"unit_price" : 1.12,
"amount" : 2.24
} ],
"deposits" : [ {
"method" : "paypal",
"amount" : 3.74,
"paid_at" : "2021-04-02T01:02:03.456Z"
} ],
"totals" : {
"sub" : 2.24,
"tax" : 1.5,
"items" : [ {
"type" : "tax",
"name" : "Tax",
"amount" : 1.5
} ],
"grand" : 3.74
}
}
}
Endpoint
POST /v2/transactions
Field | Type | Description |
---|
No query parameters.
Request
Standard post of JSON with a application/json
content type. The JSON consists of a Transaction object. See the transaction object for more details.
Response
A JSON-encoded response is returned with either an error or the new Transaction object. See the transaction object for more details.
Update Transaction
Updates an existing transaction on Greenback.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' -XPOST -d @transaction.json https://api.greenback.com/v2/transactions/e5waKyOnRv
HTTP Request
POST /v2/transactions/e5waKyOnRv HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
Content-Length: 597
Content-Type: application/json
{
"id" : "e5waKyOnRv",
"reference_id" : "113-456-71234",
"type" : "sales_receipt",
"currency_code" : "usd",
"account_id" : "za5ygRK6am",
"items" : [ {
"grn" : "it:sku:WIDGET-1",
"name" : "Widget",
"quantity" : 2.0,
"unit_price" : 1.12,
"amount" : 2.24
} ],
"deposits" : [ {
"method" : "paypal",
"amount" : 3.74,
"paid_at" : "2021-04-02T01:02:03.456Z"
} ],
"totals" : {
"sub" : 2.24,
"tax" : 1.5,
"items" : [ {
"type" : "tax",
"name" : "Tax",
"amount" : 1.5
} ],
"grand" : 3.74
},
"transacted_at" : "2021-04-01T01:02:03.456Z"
}
JSON Response
{
"value" : {
"id" : "e5waKyOnRv",
"type" : "sales_receipt",
"currency_code" : "usd",
"reference_id" : "113-456-71234",
"account_id" : "za5ygRK6am",
"transacted_at" : "2021-04-01T01:02:03.456Z",
"created_at" : "2021-04-07T04:04:09.996Z",
"updated_at" : "2021-04-07T04:04:09.998Z",
"flags" : [ "manual" ],
"items" : [ {
"grn" : "it:sku:WIDGET-1",
"name" : "Widget",
"quantity" : 2.0,
"unit_price" : 1.12,
"amount" : 2.24
} ],
"deposits" : [ {
"method" : "paypal",
"amount" : 3.74,
"paid_at" : "2021-04-02T01:02:03.456Z"
} ],
"totals" : {
"sub" : 2.24,
"tax" : 1.5,
"items" : [ {
"type" : "tax",
"name" : "Tax",
"amount" : 1.5
} ],
"grand" : 3.74
}
}
}
Endpoint
POST /v2/transactions/{transaction_id}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier for the transaction |
Request
Standard post of JSON with a application/json
content type. The JSON consists of a Transaction object. See the transaction object for more details.
Response
A JSON-encoded response is returned with either an error or the new Transaction object. See the transaction object for more details.
List Transactions
List all transactions fetched or posted to an Account sorted by default to descending (most recent) ordering.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/transactions
HTTP Request
GET /v2/transactions HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"pagination": {
"count": 5,
"total_count": 1323,
"next": "o-5,tc-1323"
},
"values": [
{
"id": "J80agMOmZ2",
"type": "purchase",
"currency_code": "aud",
"reference_id": "7SJ21259XN543224G-PPF",
"account_id": "zYP7Lv9Ea9",
"transacted_at": "2020-01-22T20:38:38.000Z",
"created_at": "2020-01-23T01:13:55.637Z",
"updated_at": "2020-01-23T01:13:55.639Z",
"attributes": {
"sale_reference_id": "7SJ21259XN543224G"
},
"meta": {
"progress": 1
},
"items": [
{
"grn": "it:vs:ppf",
"name": "Payment Processing Fees for Transaction #7SJ21259XN543224G",
"quantity": 1,
"unit_price": 1.52,
"amount": 1.52
}
],
"payments": [
{
"method": "paypal",
"sub_method": "balance",
"amount": 1.52,
"paid_at": "2020-01-22T20:38:38.000Z"
}
],
"totals": {
"sub": 1.52,
"grand": 1.52
}
},
{ ... more transactions ... }
]
}
Endpoint
GET /v2/transactions?expands={expands}&limit={limit}&cursor={cursor}&account_ids={account_ids}&&query={query}&min_transacted_at={min_transacted_at}&max_transacted_at={max_transacted_at}&min_created_at={min_created_at}&max_created_at={max_created_at}&min_updated_at={min_updated_at}&max_updated_at={max_updated_at}
Field | Type | Description |
---|---|---|
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See transaction expands for more details. |
limit | integer | (Optional) The limit for the number of transactions to return for each page of results. Defaults to 100. |
cursor | string | (Optional) The cursor for the page of transactions to return. See Pagination for more info. Defaults to none (first page of data). |
account_ids | string | (Optional) A comma-delimited list of unique account identifier of transactions to return. Defaults to none (all). |
types | enum | (Optional) One or more transaction types to filter by. A comma-delimited list such as "purchase,sales_receipt". See the Transaction object for valid enum values. Defaults to none (all) |
query | string | (Optional) A full text, search query to filter by. Any part of the structured object can be searched. For example, you can limit results to payment methods ending in last 4 digits, shipping address states, tax charged, etc. See our search help guide for more information. |
min_transacted_at | datetime | (Optional) The starting (inclusive) date and time of the transacted_at value to filter by. Defaults to none (all) |
max_transacted_at | datetime | (Optional) The ending (exclusive) date and time of the transacted_at value to filter by. Defaults to none (all) |
min_created_at | datetime | (Optional) The starting (inclusive) date and time of the created_at value to filter by. Defaults to none (all) |
max_created_at | datetime | (Optional) The ending (exclusive) date and time of the created_at value to filter by. Defaults to none (all) |
min_updated_at | datetime | (Optional) The starting (inclusive) date and time of the updated_at value to filter by. Defaults to none (all) |
max_updated_at | datetime | (Optional) The ending (exclusive) date and time of the updated_at value to filter by. Defaults to none (all) |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with a paginated list of Transaction objects. See pagination and the transaction object for more details.
Get Transaction
Get a transaction.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/transactions/z281lRLE5k
HTTP Request
GET /v2/transactions/z281lRLE5k HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value": {
"id": "z281lRLE5k",
"type": "purchase",
"currency_code": "aud",
"reference_id": "8Y109095UF481931N-PPF",
"account_id": "zYP7Lv9Ea9",
"transacted_at": "2020-01-22T10:08:54.000Z",
"created_at": "2020-01-23T01:13:55.689Z",
"updated_at": "2020-01-23T01:13:55.690Z",
"attributes": {
"sale_reference_id": "8Y109095UF481931N"
},
"meta": {
"progress": 1
},
"items": [
{
"grn": "it:vs:ppf",
"name": "Payment Processing Fees for Transaction #8Y109095UF481931N",
"quantity": 1,
"unit_price": 1.29,
"amount": 1.29
}
],
"payments": [
{
"method": "paypal",
"sub_method": "balance",
"amount": 1.29,
"paid_at": "2020-01-22T10:08:54.000Z"
}
],
"totals": {
"sub": 1.29,
"grand": 1.29
}
}
}
Endpoint
GET /v2/transactions/{transaction_id}?expands={expands}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction object. |
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See transaction expands for more details. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Transaction object. See the transaction object for more details.
Get Transaction Attachment
Get the attachment of a Transaction object by its relative unique identifier.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/transactions/kg1DMpNKXA/attachments/rs_1tydV5YSlh9bOS8fVHt9k
HTTP Request
GET /v2/transactions/kg1DMpNKXA/attachments/rs_1tydV5YSlh9bOS8fVHt9k HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
If-None-Match: 123456789456879874556fdfdf
HTTP Response
200 OK
Date: Fri, 1 Apr 2019 18:39:11 GMT
Content-Type: application/pdf
ETag: "ff12c0a4a15f17049c09054f52f14be3"
Content-Length: 29566
<binary data here>
Endpoint
GET /v2/transactions/{transaction_id}/attachments/{attachment_id}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction object. |
attachment_id | string | The relative, unique identifier of attachment. This is the rel_id value in the attachment object. |
Request
Standard GET request will contain an empty body.
Response
The binary image or document.
Export
The Export API is for exporting transactions, contacts, and other items to accounting systems (general ledgers). Exporting transactions is a complex process since it may entail matching transactions that already exist in the general ledger, or using accrual or cash accounting methods. The Export API abstracts that complexity into a simple process to present possible matches to the user, a form to ask questions about the export, and remembering choices to help speed up future exports. This generalized process works across various accounting vendors and platforms.
Get Transaction Export Intent | GET /v2/transactions/{transaction_id}/exporters/{account_id} |
Apply Transaction Export Intent | POST /v2/transactions/{transaction_id}/exporters/{account_id} |
Get Transaction Export | GET /v2/transaction_exports/{transaction_id} |
Delete Transaction Export | DELETE /v2/transaction_exports/{transaction_id} |
Export Types
Transaction Export Intent Expands
Value | Description |
---|---|
form | Includes the form for exporting the transaction. |
account | Includes the associated target accounting account. |
prefs | Includes the preferences currently configured for the target accounting account. |
matches | Includes possible transaction matches in the target accounting account. |
Transaction Export Expands
Value | Description |
---|---|
account | Includes the associated accounting account. |
targets | Includes information about the targets (what was exported) to the accounting account. For example, a deeplink URL to the entity will be generated. |
The Transaction Export Object
The Transaction Export
{
"transaction_id" : "e5waKyOnRv",
"account_id" : "oxo1glP1Yy",
"triggered_by" : "web",
"mode" : "legacy",
"accounting_method" : "cash",
"steps" : [ {
"type" : "transaction",
"action" : "create",
"source_id" : "e5waKyOnRv",
"source_amount" : 3.74,
"target_id" : "s-2400",
"target_amount" : 3.74
} ],
"created_at" : "2021-03-07T14:30:54.721Z",
"updated_at" : "2021-03-07T14:30:54.721Z"
}
The Transaction Export object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction associated with this object. |
account_id | string | The unique identifier of the Account the transaction was exported to. |
triggered_by | enum | The method used to trigger (initiate) the export. |
mode | enum | The mode used to export. See the Transaction Export Mode enum for more details. |
accounting_method | enum | The accounting method used to export. Values may include: cash , accrual , or pseudo_accrual . |
steps | object[] | An array of transaction export step objects to help track the progress of an export. See the Transaction Export Step object for more details. |
created_at | datetime | ISO 8601 timestamp of when the object was created. |
updated_at | datetime | ISO 8601 timestamp of when the object was last updated. |
The Transaction Export Step Object
The Transaction Export Step
{
"type" : "transaction",
"action" : "create",
"source_id" : "e5waKyOnRv",
"source_amount" : 3.74,
"target_id" : "s-2400",
"target_amount" : 3.74
}
The Transaction Export Step object within the Greenback API. See our reference Java implementation for more details.
Field | Type | Description |
---|---|---|
type | enum | The export type performed in this step. See the Export Type enum for more details. |
action | enum | The export action performed in this step. See the Export Action enum for more details. |
triggered_by | enum | The method used to trigger (initiate) the export. |
mode | enum | The mode used to export. See the Transaction Export Mode enum for more details. |
accounting_method | enum | The accounting method used to export. Values may include: cash , accrual , or pseudo_accrual . |
steps | object[] | An array of transaction export step objects to help track the progress of an export. See the Transaction Export Step object for more details. |
created_at | datetime | ISO 8601 timestamp of when the object was created. |
updated_at | datetime | ISO 8601 timestamp of when the object was last updated. |
The Export Type Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
transaction | A transaction was exported |
payment | The payment of a transaction was exported |
contact | A contact was exported |
The Export Action Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
create | The transaction was created by Greenback during the export |
update | The transaction was updated by Greenback during the export (it was already on your books and we updated it) |
link | The transaction was linked to an existing transaction and Greenback neither created or updated it |
pseudo | A pseudo transaction was performed (e.g. a pseudo accrual) |
none | No action was performed |
The Transaction Export Mode Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
legacy | Legacy exports treat the entire transaction as fully paid in either a single payment or deposit. |
payment | Payment exports evaluate the payments or deposits present on a transaction, supporting true accrual accounting. |
The Transaction Export Delete Mode Enum
See our reference Java implementation for more details.
Value | Description |
---|---|
default | The transaction must be deleted in the associated target accounting system before also deleted on Greenback. |
ignore_accounting_failure | Try to delete the transaction in the associated target accounting system, but ignore any failures during that delete, and then delete the export on Greenback. |
skip_accounting | Skip trying to delete in the associated target accounting system and immediately clear the export on Greenback. |
Get Transaction Export Intent
Get a transaction export intent.
Send Request
curl -v -H 'Authorization: Bearer 1234567890' https://api.greenback.com/v2/transactions/z281lRLE5k/exporters/A7zj17212d
HTTP Request
GET /v2/transactions/z281lRLE5k/exporters/A7zj17212d HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value" : {
"id" : "e5waKyOnRv",
"account" : {
"id" : "oxo1glP1Yy",
"name" : "Henry Consulting, LLC.",
"user_id" : "kNgX7qmBzR",
"connect_id" : "9ox7adX2WN",
"type" : "accounting",
"default_name" : "Henry Consulting, LLC.",
"reference_id" : "123146012333049",
"connection_state" : "ok",
"state" : "active",
"consecutive_errors" : 0,
"created_at" : "2021-04-07T05:18:09.280Z",
"updated_at" : "2021-04-07T05:18:16.068Z",
"connect" : {
"id" : "9ox7adX2WN",
"type" : "accounting",
"tags" : [ "accounting" ],
"name" : "QuickBooks Online",
"label" : "quickbooks",
"state" : "active",
"logo_url" : "https://www.greenback.com/assets/f/logos/quickbooks.intuit.com.svg",
"created_at" : "2021-04-02T23:34:10.000Z",
"updated_at" : "2021-04-02T23:34:10.000Z",
"auth_strategy" : "default"
}
},
"form" : {
"fields" : [ {
"type" : "select",
"name" : "customer",
"label" : "Customer",
"required" : true,
"options" : [ {
"value" : "c-196",
"text" : "Amazon.co.uk (United Kingdom) - Sales",
"tertiary_text" : "Customer"
}, {
"value" : "c-194",
"text" : "Amazon.com",
"tertiary_text" : "Customer"
}, {
"value" : "c-188",
"text" : "Etsy - Sales",
"tertiary_text" : "Customer"
}, {
"value" : "c-185",
"text" : "Shopify",
"tertiary_text" : "Customer"
}, {
"value" : "c-189",
"text" : "Stripe",
"tertiary_text" : "Customer"
}, {
"value" : "c-191",
"text" : "test@example.com",
"tertiary_text" : "Customer"
}, {
"value" : "c-192",
"text" : "Wix",
"tertiary_text" : "Customer"
}, {
"value" : "c-193",
"text" : "WooCommerce",
"tertiary_text" : "Customer"
} ]
}, {
"type" : "select",
"name" : "account",
"label" : "Deposit To",
"required" : true,
"options" : [ {
"value" : "",
"text" : ""
}, {
"value" : "a-1256",
"text" : "Shopify Payments",
"tertiary_text" : "Bank"
}, {
"value" : "a-1264",
"text" : "Inventory Asset",
"tertiary_text" : "Other Current Asset"
}, {
"value" : "a-1220",
"text" : "Prepaid Expenses",
"tertiary_text" : "Other Current Asset"
}, {
"value" : "a-1254",
"text" : "Uncategorized Asset",
"tertiary_text" : "Other Current Asset"
}, {
"value" : "a-1221",
"text" : "Undeposited Funds",
"tertiary_text" : "Other Current Asset"
} ],
"help" : "Funds deposited to an unspecified PayPal"
}, {
"type" : "select",
"name" : "lines[0].category",
"label" : "Product/Service #1",
"required" : true,
"options" : [ {
"value" : "",
"text" : ""
}, {
"value" : "item-89",
"text" : "Hours",
"tertiary_text" : "Product/Service"
}, {
"value" : "item-88",
"text" : "Services",
"tertiary_text" : "Product/Service"
} ],
"help" : "Widget"
}, {
"type" : "select",
"name" : "tax",
"label" : "Tax",
"required" : true,
"options" : [ {
"value" : "",
"text" : ""
}, {
"value" : "taxcode-NOT_APPLICABLE_TAX_CODE",
"text" : "Not Applicable (distribute across line items)"
}, {
"value" : "taxcode-30",
"text" : "MI (6%)"
}, {
"value" : "taxcode-29",
"text" : "Out of scope (0%)"
} ],
"help" : "Estimated tax rate is 67%"
}, {
"type" : "select",
"name" : "department",
"label" : "Location (Optional)",
"required" : false,
"options" : [ {
"value" : "",
"text" : ""
} ]
}, {
"type" : "select",
"name" : "clazz",
"label" : "Class (Optional)",
"required" : false,
"options" : [ {
"value" : "",
"text" : ""
} ]
} ]
}
}
}
Endpoint
GET /v2/transactions/{transaction_id}/exporters/{account_id}?expands={expands}&itemized={itemized}&verified_by={verified_by}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction object. |
account_id | string | The unique identifier of the Account object you want to export to. The account must be of an accounting type. |
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See transaction export intent expands for more details. |
itemized | boolean | (Optional) Whether summary or itemized mode should be set and override the accounting preferences currently set. |
verified_by | datetime | (Highly Recommended) An ISO timestamp of what point-in-time should the target accounting system have its data verified to. This timestamp is critical for performance during exporting, so we suggest you include it. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Transaction Exporter object. See the transaction exporter object for more details.
Apply Transaction Export Intent
Export a transaction to an accounting (general ledger) and get back a Transaction Export object.
HTTP Request
POST /v2/transactions/z281lRLE5k/exporters/A7zj17212d HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
Content-Type: application/json
Content-Length: 161
{
"parameters" : {
"customer" : "c-194",
"account" : "a-1256",
"lines[0].category" : "item-89",
"tax" : "taxcode-NOT_APPLICABLE_TAX_CODE"
}
}
JSON Response
{
"value" : {
"transaction_id" : "e5waKyOnRv",
"account_id" : "oxo1glP1Yy",
"triggered_by" : "web",
"mode" : "legacy",
"accounting_method" : "cash",
"steps" : [ {
"type" : "transaction",
"action" : "create",
"source_id" : "e5waKyOnRv",
"source_amount" : 3.74,
"target_id" : "s-2400",
"target_amount" : 3.74
} ],
"created_at" : "2021-04-07T14:30:54.721Z",
"updated_at" : "2021-04-07T14:30:54.721Z"
}
}
Endpoint
POST /v2/transactions/{transaction_id}/exporters/{account_id}?itemized={itemized}&verified_by={verified_by}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction object. |
account_id | string | The unique identifier of the Account object you want to export to. The account must be of an accounting type. |
itemized | boolean | (Optional) Whether summary or itemized mode should be set and override the accounting preferences currently set. |
verified_by | datetime | (Highly Recommended) An ISO timestamp of what point-in-time should the target accounting system have its data verified to. This timestamp is critical for performance during exporting, so we suggest you include it. |
Request
Standard POST request will contain a body of application/json
Field | Type | Description |
---|---|---|
parameters | map | A map of key-value string pairs that supplies the answers to the "form" returned in the Get Transaction Exporter response. |
Response
A JSON-encoded response is returned with either an error or a Transaction Export object. See the transaction export object for more details.
Get Transaction Export
Gets a Transaction Export.
HTTP Request
GET /v2/transaction_exports/z281lRLE5k HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value" : {
"transaction_id" : "z281lRLE5k",
"account_id" : "oxo1glP1Yy",
"triggered_by" : "web",
"mode" : "payment",
"accounting_method" : "cash",
"steps" : [ {
"type" : "transaction",
"action" : "create",
"source_id" : "e5waKyOnRv",
"source_amount" : 3.74,
"target_id" : "s-2400",
"target_amount" : 3.74
} ],
"created_at" : "2021-04-07T14:30:54.721Z",
"updated_at" : "2021-04-07T14:30:54.721Z"
}
}
Endpoint
GET /v2/transaction_exports/{transaction_id}?expands={expands}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction object. |
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See transaction export intent expands for more details. |
Request
Standard GET request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Transaction Export object. See the transaction export object for more details.
Delete Transaction Export
Deletes a Transaction Export.
HTTP Request
DELETE /v2/transaction_exports/z281lRLE5k HTTP/1.1
Host: api.greenback.com
Authorization: Bearer 1234567890
Accept: application/json
JSON Response
{
"value" : {
"transaction_id" : "z281lRLE5k",
"account_id" : "oxo1glP1Yy",
"triggered_by" : "web",
"mode" : "payment",
"accounting_method" : "cash",
"steps" : [ {
"type" : "transaction",
"action" : "create",
"source_id" : "e5waKyOnRv",
"source_amount" : 3.74,
"target_id" : "s-2400",
"target_amount" : 3.74
} ],
"created_at" : "2021-04-07T14:30:54.721Z",
"updated_at" : "2021-04-07T14:30:54.721Z"
}
}
Endpoint
DELETE /v2/transaction_exports/{transaction_id}?expands={expands}&mode={mode}
Field | Type | Description |
---|---|---|
transaction_id | string | The unique identifier of the Transaction object. |
expands | string | (Optional) Additional associated objects to expand as part of the response. A comma-delimited list of expansions. See transaction export intent expands for more details. |
mode | enum | (Optional) The mode to use for the delete (which controls what Greenback tries to do with the export in the target accounting system) See transaction export delete mode enum for more details. |
Request
Standard DELETE request will contain an empty body.
Response
A JSON-encoded response is returned with either an error or a Transaction Export object. See the transaction export object for more details.